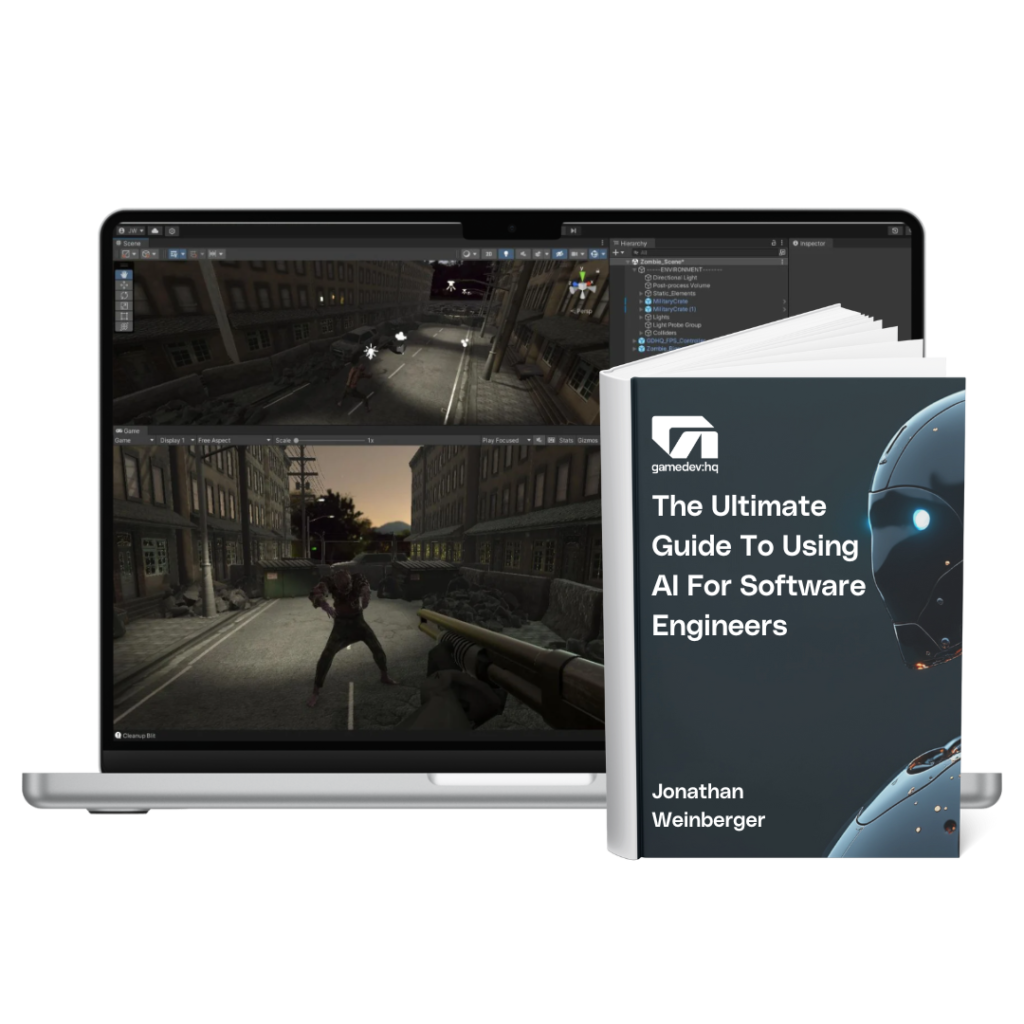
Understanding char in C#: A Building Block of Text and Characters
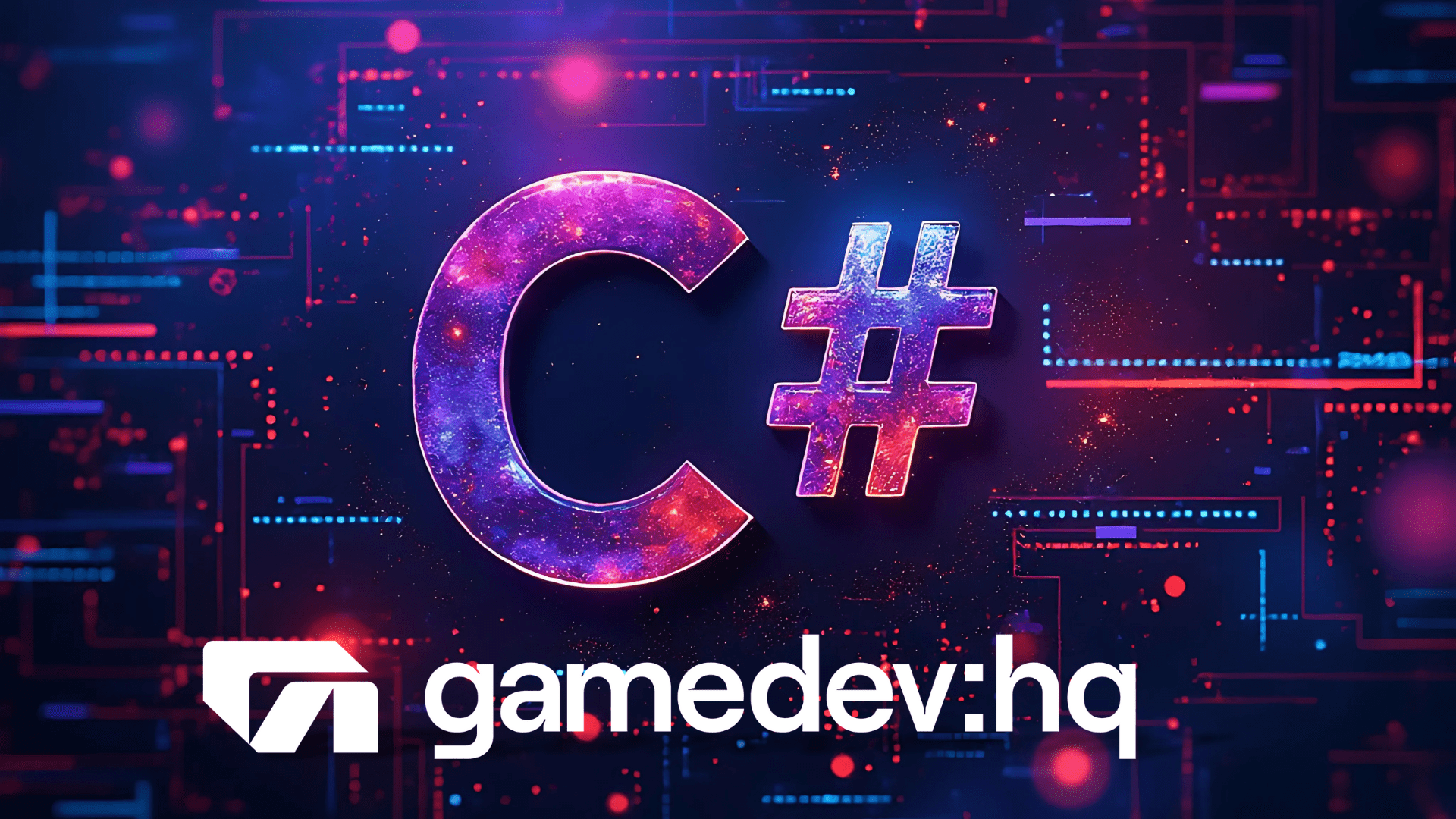
When we think about text and characters in programming, the char
data type in C# serves as a fundamental building block. Much like individual bricks in a wall, each char
is a single character that contributes to forming strings, which are sequences of characters. Just as a wall is only as strong as its individual bricks, understanding how to effectively use char
can enhance your coding prowess, especially in areas like game development where text manipulation is often required.
What is a char
?
In C#, a char
is a data type used to store a single 16-bit Unicode character. This means it can represent any character in the Unicode standard, making it versatile for various languages and symbols. It occupies two bytes in memory and can hold a value ranging from \u0000
to \uFFFF
.
Sample Code Uses of char
1. Simple Character Assignment
// Declaration and initialization of a char
char letter = 'A';
// Display the char
Console.WriteLine("The character is: " + letter);
In this basic example, we declare a char
variable named letter
and initialize it with the character ‘A’. We then print this character to the console.
2. Character Manipulation
// Converting to uppercase
char lowercase = 'b';
char uppercase = Char.ToUpper(lowercase);
Console.WriteLine("Uppercase of " + lowercase + " is: " + uppercase);
Here, we demonstrate character manipulation by converting a lowercase character to uppercase using the Char.ToUpper()
method. This is particularly useful in scenarios where case sensitivity matters, such as usernames or codes.
3. Game Development Scenario: Checking Player Input
// Checking key press in a game
char keyPressed = 'W'; // Imagine this is received from player input
if (keyPressed == 'W' || keyPressed == 'w')
{
Console.WriteLine("Move character forward.");
}
else if (keyPressed == 'S' || keyPressed == 's')
{
Console.WriteLine("Move character backward.");
}
In a gaming context, detecting player inputs is crucial. Here, we use char
to handle player commands for movement. The code checks if the player pressed ‘W’ or ‘w’ to move forward, demonstrating a simple use of char
in a conditional statement.
A char
in C# is a versatile tool that can control text output, manage user input, and even play a crucial role in game development. By mastering char
, developers can enhance the interactivity and functionality of their software, much like a skilled mason uses bricks to construct a masterpiece. Whether you’re building a text-based interface or developing a game, understanding and utilizing char
effectively is essential.