How to Calculate Direction in Unity – A Unity Math Tutorial
Ever wondered how zombies in games always know where to find you? It's not magic - it's math!
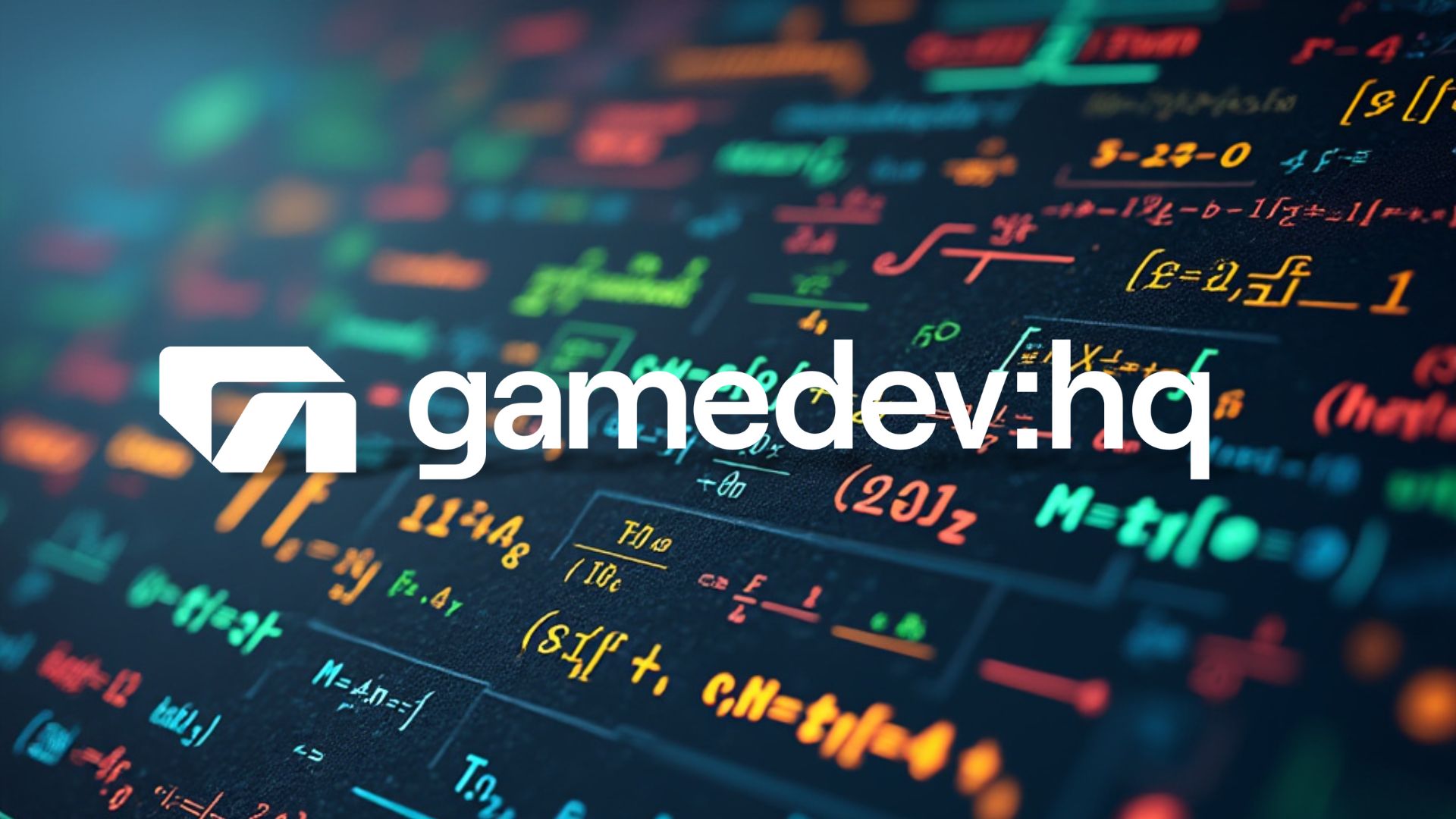
Ever wondered how zombies in games always know where to find you? It’s not magic – it’s math! As a game developer who once struggled with numbers, I’ve discovered that math becomes way more interesting when you apply it to game development. Let’s dive into how we calculate direction in Unity, a skill crucial for creating engaging enemy AI in games like first-person shooters.
The Myth of Math Mastery
Many aspiring game developers believe they need to be math geniuses to succeed. That’s not true! I failed math throughout school, but when I started making games, suddenly those abstract concepts became practical tools. It’s like learning to use a sword in an RPG – at first, it seems complicated, but with practice, it becomes second nature.
The Magic Triangle
Imagine you’re making a zombie game. Your player is at position (4, 0), and a zombie is at (0, 8). To an observant eye, these points form a right-angled triangle. This triangle is the key to understanding direction and distance in game development.Remember the Pythagorean theorem? It’s not just a school memory – it’s the foundation of our direction calculations! In our zombie game, it helps us determine how far the zombie needs to travel to reach the player.
Direction Formula Unveiled
Here’s the simple yet powerful direction formula:Direction = Destination – SourceIn game terms:
- Destination: Player’s position
- Source: Enemy’s position
This calculation gives us a vector – imagine it as an arrow pointing from the zombie to the player.
Coding Direction in Unity
Let’s implement this in Unity:
- Create a new C# script called “CalculateDirection” and attach it to our enemy.
- Add a reference to the player:
csharp[SerializeField]
private Transform player;
- Calculate the direction:
csharpVector3 direction = player.position - transform.position;
- Visualize the direction:
csharpDebug.DrawRay(transform.position, direction, Color.green);
- Get the distance:
csharpfloat distance = direction.magnitude;
Debug.Log("Distance: " + distance);
Moving the Enemy
Now that we have direction, let’s make our zombie move:
csharptransform.Translate(direction.normalized * Time.deltaTime * speed);
We normalize the direction to ensure consistent movement speed, regardless of distance.
The Power of Math in Games
Just like how leveling up in an RPG makes your character stronger, understanding these math concepts empowers you as a game developer. The Pythagorean theorem, once a dreaded exam topic, becomes a tool for creating thrilling chase sequences in your games.Next time you’re playing a game and an enemy starts chasing you, remember – it’s not just following you, it’s using math to calculate its path. And now, you know how to create that behavior yourself!By mastering these concepts, you’re not just learning math – you’re learning to create more engaging and realistic game worlds. So embrace the math, and watch your games come to life!