Learn to Program with C# – CONSTRUCTORS – Intermediate Unity Tutorial
Let's jump into the world of constructors and see how they can enhance your game development process.
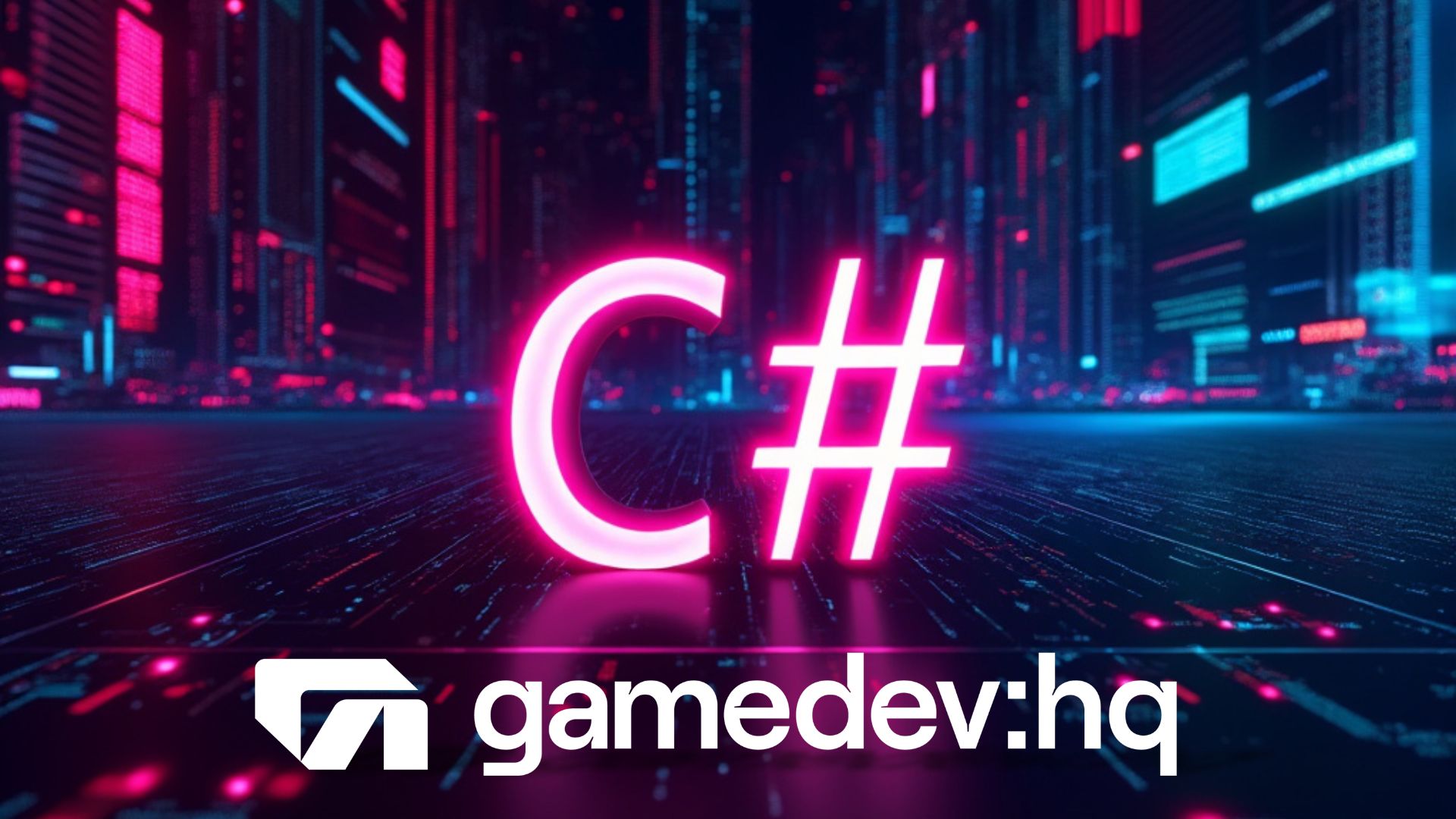
Let’s jump into the world of constructors and see how they can enhance your game development process.
What are Constructors?
Constructors are special methods in C# that are automatically called when you create a new instance of a class. They’re like the character creation screen in an RPG – they set up the initial state of your objects.
In game development, constructors are particularly useful for initializing game objects, such as items, characters, or levels. For example, in a first-person shooter (FPS), you might use constructors to create different types of weapons with specific attributes.
Creating a Basic Constructor
Let’s start by creating a simple Weapon
class for our FPS game:
public class Weapon
{
public int id;
public string name;
public int damage;
public Weapon(int weaponId, string weaponName, int weaponDamage)
{
id = weaponId;
name = weaponName;
damage = weaponDamage;
}
}
In this example, the constructor takes three parameters to initialize the weapon’s properties. When we create a new weapon, we can set its attributes immediately:
Weapon pistol = new Weapon(1, "Pistol", 10);
Overloading Constructors
Sometimes, we might want to create objects with different sets of initial properties. This is where constructor overloading comes in handy. We can define multiple constructors with different parameters:
public class Weapon
{
public int id;
public string name;
public int damage;
public int ammo;
public Weapon(int weaponId, string weaponName, int weaponDamage)
{
id = weaponId;
name = weaponName;
damage = weaponDamage;
ammo = 0;
}
public Weapon(int weaponId, string weaponName, int weaponDamage, int weaponAmmo)
{
id = weaponId;
name = weaponName;
damage = weaponDamage;
ammo = weaponAmmo;
}
}
Now we can create weapons with or without specifying the initial ammo:
Weapon pistol = new Weapon(1, "Pistol", 10);
Weapon rifle = new Weapon(2, "Rifle", 20, 30);
Using Constructors with Unity MonoBehaviour
When working with Unity, remember that MonoBehaviour classes don’t use traditional constructors. Instead, you’ll typically use the Awake()
or Start()
methods to initialize your objects:
public class WeaponBehaviour : MonoBehaviour
{
public int id;
public string weaponName;
public int damage;
private void Awake()
{
InitializeWeapon(1, "Default Weapon", 10);
}
public void InitializeWeapon(int weaponId, string name, int weaponDamage)
{
id = weaponId;
weaponName = name;
damage = weaponDamage;
}
}
Practical Examples in Game Development
Let’s look at some practical examples of using constructors in game development:
Item System
public class Item
{
public int id;
public string name;
public string description;
public Item(int itemId, string itemName)
{
id = itemId;
name = itemName;
description = "";
}
public Item(int itemId, string itemName, string itemDescription)
{
id = itemId;
name = itemName;
description = itemDescription;
}
}
This Item
class allows us to create items with or without a description.
Character Creation
public class Character
{
public string name;
public int health;
public int level;
public Character(string characterName)
{
name = characterName;
health = 100;
level = 1;
}
public Character(string characterName, int startingHealth, int startingLevel)
{
name = characterName;
health = startingHealth;
level = startingLevel;
}
}
This Character
class allows for creating new characters with default values or custom starting attributes.
Best Practices for Using Constructors
- Keep it Simple: Constructors should primarily initialize object state. Avoid complex logic or operations.
- Use Overloading Wisely: Provide overloaded constructors when it makes sense, but don’t go overboard.
- Consider Default Values: Use default parameters or separate constructors for optional values.
- Validate Input: If necessary, add input validation in your constructors to ensure object integrity.
- Use Properties: For more complex initialization, consider using properties and initialization methods instead of cramming everything into the constructor.
FAQs
Q: Can I call one constructor from another in C#?
A: Yes, you can use the this
keyword to call another constructor within the same class. This is known as constructor chaining.
Q: Are constructors inherited in C#?
A: Constructors are not inherited, but a derived class can call a base class constructor using the base
keyword.
Q: Can a constructor be private?
A: Yes, private constructors are used to prevent direct instantiation of a class, often in singleton patterns or static utility classes.
Q: How do constructors work with Unity’s serialization?
A: Unity’s serialization system doesn’t use constructors. For MonoBehaviour classes, use Awake()
or Start()
for initialization.
By mastering constructors, you’ll be able to create more organized and efficient code for your games. Whether you’re developing an FPS, RPG, or any other genre, constructors provide a solid foundation for building complex game systems. Happy coding!