Understanding Static Types in C#: A Unity Tutorial
Imagine you need a global inventory system that every character can access. This is where static types in C# come into play, offering a unique way to manage shared resources across your game's universe.
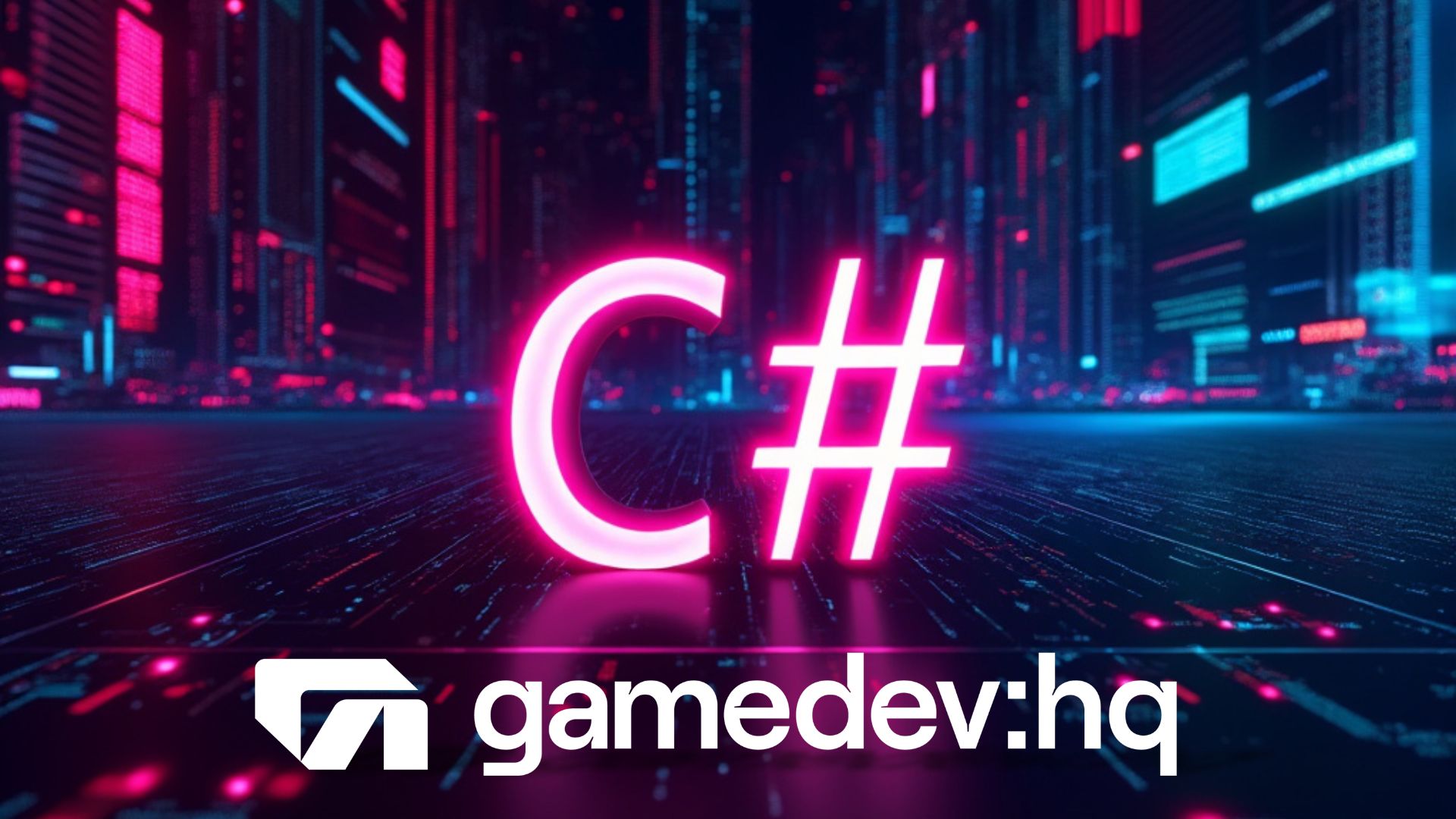
Imagine you’re designing a role-playing game (RPG) where you need a global inventory system that every character can access. This is where static types in C# come into play, offering a unique way to manage shared resources across your game’s universe. Let’s explore the concept of static types, their benefits, and potential pitfalls, much like balancing the mechanics of an RPG.
What Are Static Types?
In C#, the static
keyword can be applied to classes, variables, and functions. When something is static, it belongs to the type itself rather than any instance of the type. This means you can access it without creating an object of that class. It’s akin to having a shared inventory in an RPG that all characters can access without needing their own personal copy.
Pros and Cons of Static Types
Pros
- Global Access: Static types provide easy access to variables and methods from anywhere in your code. This is like having a universal spellbook in your RPG that any character can read from at any time.
- Singleton Pattern: Static types are often used in design patterns like singletons, where you need only one instance of a class throughout your application. Imagine having one guild master in your RPG who manages all guild-related activities.
- Utility and Helper Classes: Static methods are perfect for utility functions that don’t rely on object state. Think of them as universal potions that heal any character without needing to be part of their personal inventory.
Cons
- Memory Usage: Static variables occupy memory for the lifetime of the application, which can lead to inefficient memory usage if not managed properly. It’s like hoarding items in your RPG inventory that you never use, taking up valuable space.
- No Garbage Collection: Since static members are not tied to an instance, they aren’t cleaned up by garbage collection until the program ends. This can be problematic in resource-constrained environments like mobile games.
- Limited Flexibility: Static classes cannot be instantiated or inherited, which limits their flexibility compared to regular classes. It’s like having a legendary sword in your RPG that can’t be upgraded or customized.
Practical Uses of Static Types
Manager Classes
In game development, manager classes often use static variables and methods to handle global states or resources. For example, a GameManager
might track the current level or score using static fields, ensuring consistent data across scenes.
public static class GameManager
{
public static int CurrentLevel { get; set; }
public static int Score { get; set; }
}
Utility Functions
Static methods are ideal for utility functions that perform common tasks across different parts of your game. For instance, converting world coordinates to screen coordinates might be a static method in a MathUtils
class.
public static class MathUtils
{
public static Vector3 WorldToScreen(Vector3 worldPosition)
{
return Camera.main.WorldToScreenPoint(worldPosition);
}
}
When Not to Use Static Types
Avoid using static variables for data that changes frequently or is specific to an instance of a class. In our RPG analogy, this would be like trying to store each character’s health as a static variable—each character needs their own health value!
Best Practices for Using Static Types
- Use Sparingly: Only use static types when truly necessary, such as for constants or utility functions.
- Consider Memory Impact: Be mindful of the memory implications, especially in environments with limited resources.
- Design Patterns: Utilize design patterns like singletons wisely to manage global states without excessive memory use.
- Testing: Ensure thorough testing when using static types, as they can introduce hard-to-track bugs due to their global nature.
FAQs
What is a static class?
A static class is a class that cannot be instantiated or inherited from. It is used primarily for grouping related methods and properties that do not need an object context.
Can I change a static variable during runtime?
Yes, you can change the value of a static variable during runtime; however, remember that it affects all parts of your program accessing it since there is only one copy.
How does garbage collection work with statics?
Garbage collection does not automatically clean up static variables because they are tied to the application’s lifetime rather than individual objects.
Are there alternatives to using statics?
Yes, consider using dependency injection or singleton patterns with care if you need shared resources without resorting to statics.
Conclusion
Static types in C# offer powerful tools for managing shared resources and utilities within Unity projects. Like managing shared resources in an RPG, they require careful consideration and strategic use to avoid pitfalls such as excessive memory usage and inflexibility. By understanding when and how to use statics effectively, you can enhance your game’s architecture while maintaining performance and clarity.