Learn to Program with C# – What’s an Array in C Sharp? – Unity Tutorial
Arrays are like magical backpacks in our RPG. Instead of carrying individual items separately, an array allows us to group similar items together in one container.
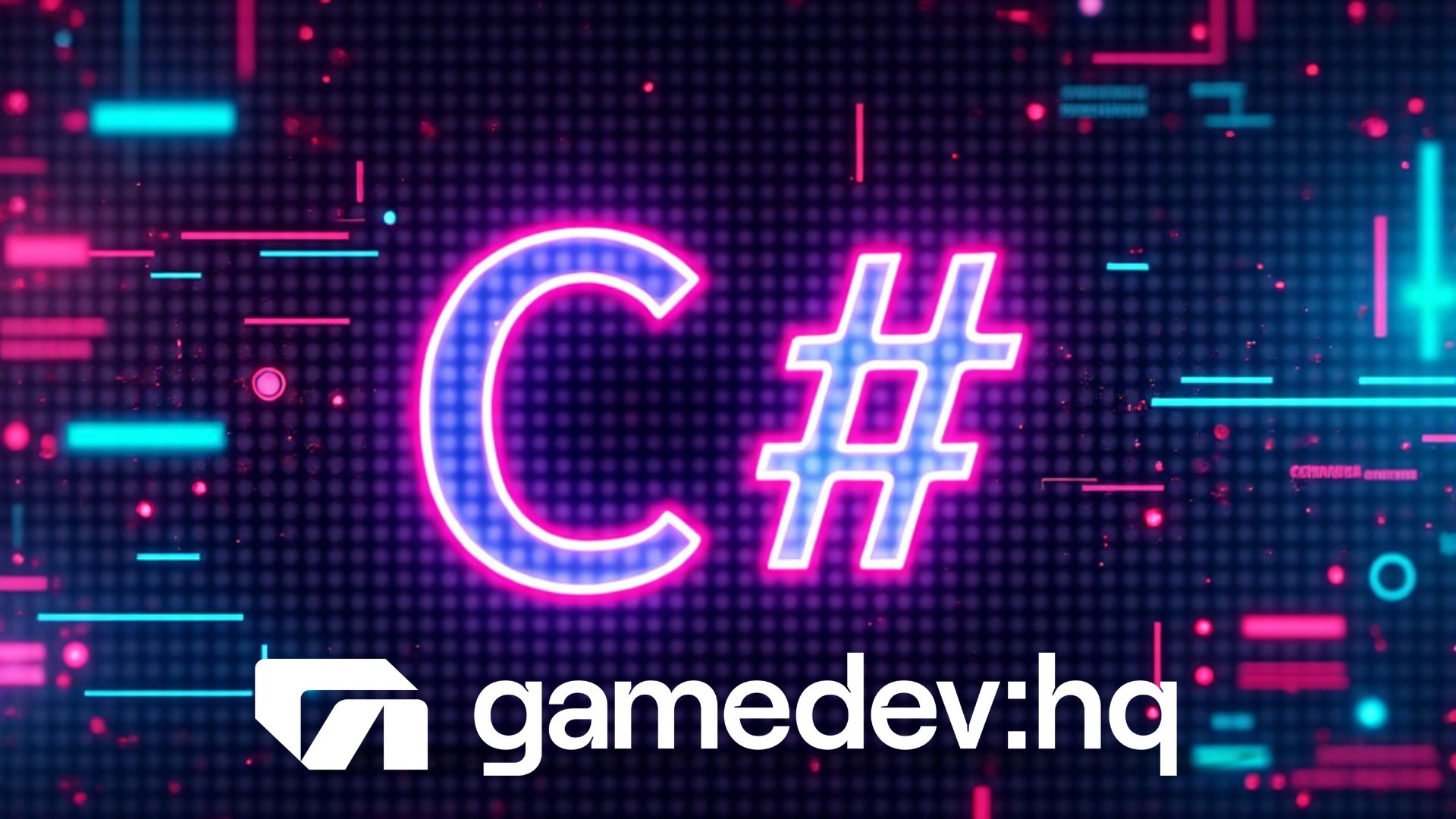
Diving into the world of arrays is like unlocking a treasure chest of possibilities in game development. Let me take you on a journey through the realm of arrays, using the analogy of creating an inventory system for a role-playing game (RPG).
What Are Arrays?
Arrays are like magical backpacks in our RPG. Instead of carrying individual items separately, an array allows us to group similar items together in one container. It’s a variable that can store multiple values of the same data type.
Imagine you’re creating an inventory system for your hero. Instead of declaring separate variables for each item, you can use an array to store all the items in one place.
public string[] inventoryItems = { "Sword", "Shield", "Potion", "Map" };
This array, inventoryItems
, now holds four string values representing different items our hero carries.
Declaring Arrays
There are multiple ways to declare arrays in C#, each with its own use case:
- Initializing with values:
public int[] playerLevels = { 1, 5, 10, 15, 20 };
This is like pre-filling our hero’s experience levels for different skills.
- Declaring with a specific size:
public float[] enemyHealths = new float[5];
Here, we’re creating space for 5 enemy health values, but we haven’t assigned them yet.
- Unity Inspector method:
public GameObject[] NPCs;
This allows us to drag and drop game objects in the Unity Inspector, like assigning different NPC characters to our game world.
Accessing Array Elements
Array elements are accessed using an index, starting from 0. It’s like numbering the pockets in our magical backpack.
Debug.Log("First item in inventory: " + inventoryItems[0]);
// Output: First item in inventory: Sword
Remember, the last element’s index is always one less than the array’s length. For our inventoryItems
array with 4 items, the last index is 3.
Array Length
To find out how many items are in our array:
int itemCount = inventoryItems.Length;
Debug.Log("Number of items: " + itemCount);
// Output: Number of items: 4
This is crucial when you want to loop through all items or check if the inventory is full.
Modifying Array Elements
You can change array elements after declaration:
inventoryItems[2] = "Elixir";
// Now the third item (index 2) is "Elixir" instead of "Potion"
Looping Through Arrays
Often, you’ll want to perform actions on all elements of an array. This is where loops come in handy:
for (int i = 0; i < inventoryItems.Length; i++)
{
Debug.Log("Item " + (i + 1) + ": " + inventoryItems[i]);
}
This loop will display all items in our inventory.
Multidimensional Arrays
Sometimes, you need more complex data structures. Multidimensional arrays are like creating a grid or table:
int[,] mapGrid = new int[3, 3] {
{0, 1, 0},
{1, 1, 1},
{0, 1, 0}
};
This could represent a simple map layout where 1 is a path and 0 is an obstacle.
Jagged Arrays
Jagged arrays are arrays of arrays, useful for storing data with varying lengths:
string[][] questDialogue = new string[3][];
questDialogue[0] = new string[] { "Hello, adventurer!", "Are you ready for a quest?" };
questDialogue[1] = new string[] { "The dragon awaits." };
questDialogue[2] = new string[] { "You've returned!", "Was your journey successful?", "Here's your reward." };
This structure allows different NPCs to have varying amounts of dialogue.
Arrays vs Lists
While arrays are powerful, they have limitations. Their size is fixed once declared. For more flexibility, you might want to use Lists, which we’ll cover in more advanced tutorials.
Practical Applications in Game Development
- Inventory Systems: Store and manage player items.
- Enemy Spawning: Keep track of multiple enemies.
- Dialogue Systems: Manage conversation options.
- Level Design: Store level layouts or object placements.
- Skill Trees: Manage player abilities and upgrades.
FAQs
- Q: Why do array indices start at 0?
A: It’s a convention that stems from how computer memory is addressed. The first element is at the starting memory location of the array. - Q: Can I mix different data types in an array?
A: No, all elements in a C# array must be of the same type. For mixed types, consider using a List <object> or creating a custom class. - Q: How do I add or remove elements from an array?
A: Arrays have a fixed size. To add or remove elements, you’d need to create a new array. This is why Lists are often preferred for dynamic collections. - Q: Are there performance considerations when using large arrays?
A: Large arrays can consume significant memory. For very large datasets, consider using more efficient data structures or database solutions. - Q: Can I use arrays with Unity’s serialization?
A: Yes, arrays work well with Unity’s serialization, allowing you to easily expose them in the Inspector for editing.
Arrays are fundamental building blocks in programming and game development. Mastering them opens up a world of possibilities for organizing and manipulating data in your games. As you continue your journey in game development, you’ll find countless creative ways to use arrays to bring your virtual worlds to life.