Learn to Program with C# – NAMESPACES – Unity Tutorial
Understanding namespaces in C# is like organizing your toolbox for building the perfect first-person shooter.
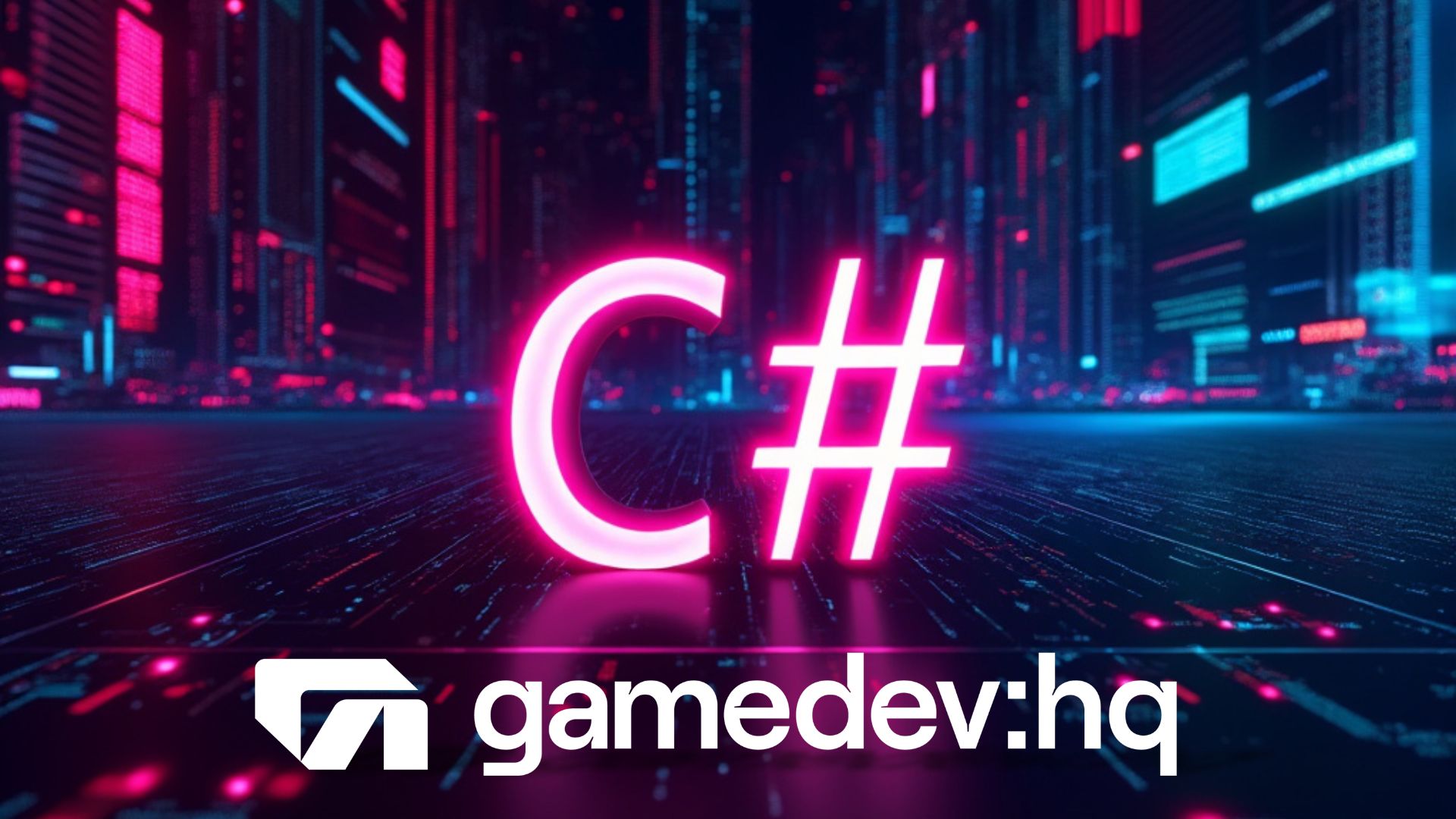
As a game developer, I’ve found that understanding namespaces in C# is like organizing your toolbox for building the perfect first-person shooter. Let me walk you through the concept and show you how to wield this powerful organizational tool in your Unity projects.
What Are Namespaces?
Namespaces in C# are like the different compartments in your FPS weapon loadout. They help you organize and categorize your code, making it easier to manage and avoid conflicts. Just as you wouldn’t want your sniper rifle attachments mixed up with your shotgun shells in your FPS, namespaces keep your code elements neatly separated.
In Unity, we’re already familiar with namespaces like UnityEngine
, which gives us access to essential game development tools. These are the pre-loaded weapons in our arsenal, ready for use in any project.
Creating Your Own Namespace
While it’s not always necessary to create your own namespaces, knowing how to do so can be valuable for larger projects or when you’re developing reusable code libraries. Here’s how you can create a custom namespace:
namespace Helper
{
public class Position
{
public static Vector3 SetPositionToZero()
{
return Vector3.zero;
}
}
}
In this example, we’ve created a Helper
namespace containing a Position
class with a static method SetPositionToZero()
. This is like creating a custom weapon mod for your FPS game – it’s a specialized tool that you can use across different parts of your project.
Using Your Custom Namespace
To use your custom namespace in another script, you need to “equip” it, just like selecting a weapon in your loadout. You do this by adding a using
statement at the top of your script:
using Helper;
public class Player : MonoBehaviour
{
void Start()
{
transform.position = Position.SetPositionToZero();
}
}
By adding using Helper;
, you now have direct access to the Position
class and its methods. This is similar to how in an FPS game, selecting a weapon gives you immediate access to its functions without having to navigate through menus.
The Static Class Alternative
While namespaces are powerful, for smaller projects or utility functions, a static class can be a more straightforward approach. It’s like having a multi-tool in your FPS loadout – always accessible and doesn’t require any special setup.
Here’s how you can create a static utility class:
public static class Utilities
{
public static Vector3 SetPositionToZero()
{
return Vector3.zero;
}
}
You can then use this anywhere in your project without needing to import a namespace:
public class Player : MonoBehaviour
{
void Start()
{
transform.position = Utilities.SetPositionToZero();
}
}
This approach is often preferred for helper functions as it’s simpler and doesn’t require managing additional namespaces.
Extending Existing Classes
One powerful feature in C# is the ability to extend existing classes with new methods. This is like adding a new firing mode to an existing weapon in your FPS game. Here’s an example of how to extend the Transform
class:
public static class Utilities
{
public static Vector3 SetPositionToZero(this Transform trans)
{
trans.position = Vector3.zero;
return trans.position;
}
}
Now you can use this method directly on any Transform
component:
transform.SetPositionToZero();
This extension method appears as if it were a built-in method of the Transform
class, making your code more intuitive and easier to read.
Best Practices
- Use namespaces for large libraries: If you’re creating a substantial codebase that might be reused across projects, namespaces can help organize your code effectively.
- Prefer static classes for small utility functions: For simple helper methods, a static class is often more straightforward and doesn’t require importing a namespace.
- Be cautious with extension methods: While powerful, overusing extension methods can make your code harder to understand for other developers. Use them judiciously.
- Keep your namespaces organized: Just as you’d organize your FPS loadout for quick access, keep your namespaces logically structured.
- Avoid namespace conflicts: Ensure your custom namespaces don’t clash with existing ones, just as you wouldn’t want two weapons with the same name in your FPS game.
FAQs
Q: When should I use namespaces instead of static classes?
A: Use namespaces when you have a large set of related classes and methods that form a cohesive library. Static classes are better for smaller sets of utility functions.
Q: Can I nest namespaces?
A: Yes, you can nest namespaces to create a hierarchy, similar to how you might have categories and subcategories of weapons in an FPS game.
Q: Do namespaces affect performance?
A: No, namespaces are a compile-time feature and do not impact runtime performance.
Q: How do I resolve naming conflicts between namespaces?
A: You can use fully qualified names (e.g., MyNamespace.MyClass
) or create aliases using the using
directive to resolve conflicts.
In conclusion, whether you choose to use namespaces or static classes, the key is to organize your code in a way that makes sense for your project. Just as a well-organized loadout can make you a more effective FPS player, well-structured code can make you a more efficient developer. Keep practicing, and soon you’ll be wielding namespaces and static classes with the same precision as a skilled marksman in your favorite shooter game.