Learn to Program with C# – ENUMS – Advanced Unity Tutorial
As a game developer, I've found enums incredibly useful for managing states and creating more organized code. Let's break this down in a way that's easy to understand, using some fun game development examples along the way.
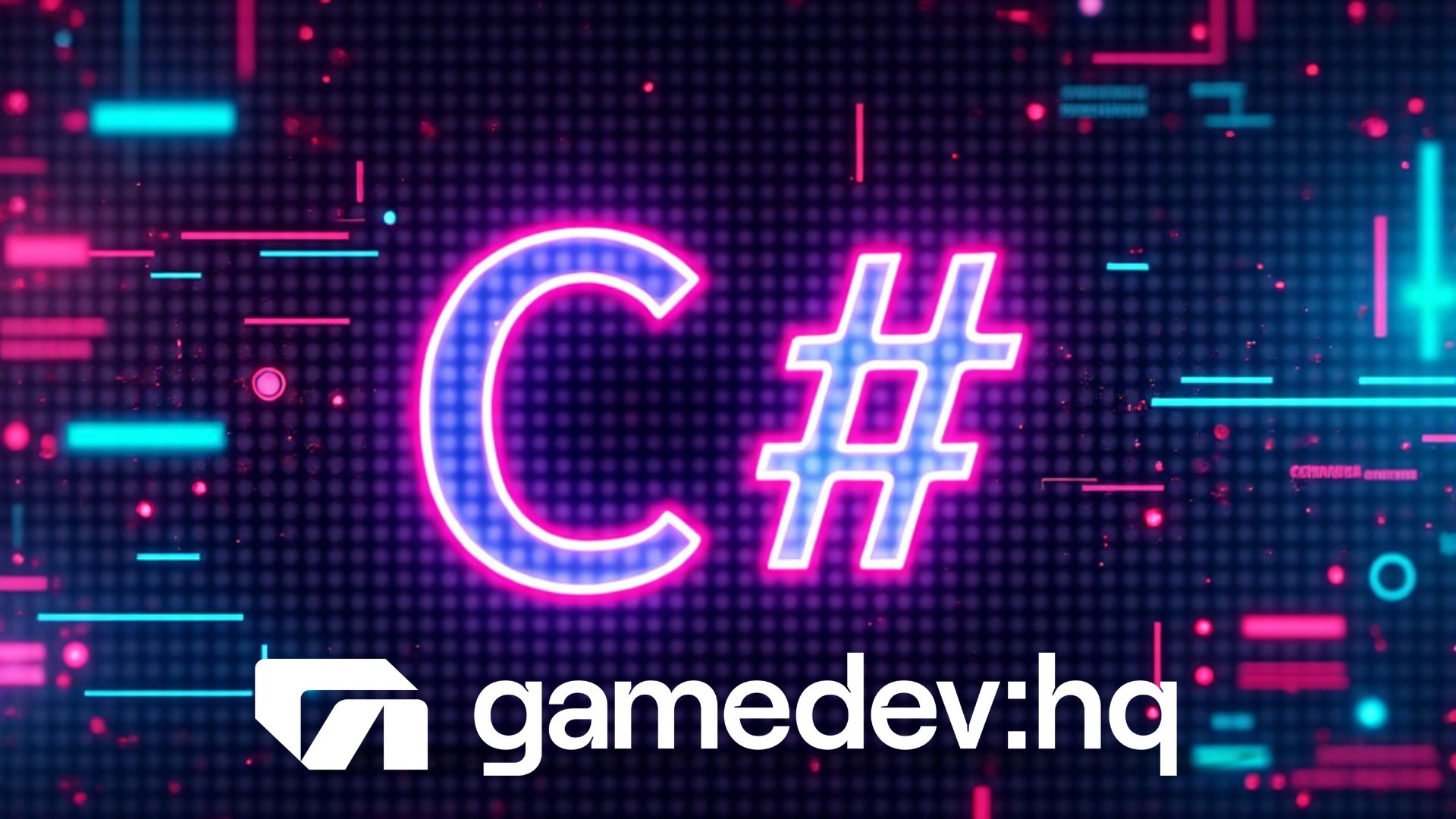
Hey there! I’m excited to dive into the world of enums in C# and Unity. As a game developer, I’ve found enums incredibly useful for managing states and creating more organized code. Let’s break this down in a way that’s easy to understand, using some fun game development examples along the way.
What Are Enums?
Enums, short for enumerations, are a powerful tool in C# that allow us to define a set of named constants. Think of them as a predefined list of options that we can use in our code. In game development, enums are particularly useful for representing different states or types within our game.
Imagine we’re creating a first-person shooter (FPS) game. We might use enums to represent different weapon types, player states, or enemy behaviors. For example:
public enum WeaponType
{
Pistol,
Shotgun,
AssaultRifle,
SniperRifle
}
This enum defines four different weapon types that our player can use. It’s much cleaner and more intuitive than using integer values to represent these types.
How to Declare and Use Enums
Declaring Enums
To declare an enum, we use the enum
keyword followed by the name of the enum. Here’s a basic structure:
public enum EnumName
{
Value1,
Value2,
Value3
}
In our FPS game, we might declare an enum for player states:
public enum PlayerState
{
Idle,
Running,
Jumping,
Crouching,
Shooting
}
Using Enums
Once we’ve declared an enum, we can use it as a type for variables. For example:
public class Player : MonoBehaviour
{
public PlayerState currentState;
void Start()
{
currentState = PlayerState.Idle;
}
}
Now, in the Unity Inspector, we’ll see a dropdown menu for the currentState
variable, allowing us to easily select from our defined states.
Enums in Action: A Simple State Machine
Let’s create a basic state machine for an enemy in our FPS game using enums. This is a common use case in game AI.
public class Enemy : MonoBehaviour
{
public enum EnemyState
{
Patrolling,
Chasing,
Attacking,
Fleeing
}
public EnemyState currentState;
void Update()
{
switch (currentState)
{
case EnemyState.Patrolling:
Patrol();
break;
case EnemyState.Chasing:
ChasePlayer();
break;
case EnemyState.Attacking:
AttackPlayer();
break;
case EnemyState.Fleeing:
Flee();
break;
}
}
void Patrol() { /* Patrol logic */ }
void ChasePlayer() { /* Chase logic */ }
void AttackPlayer() { /* Attack logic */ }
void Flee() { /* Flee logic */ }
}
This setup allows us to easily manage different behaviors for our enemy based on its current state.
Advanced Enum Techniques
Enum with Flags
Sometimes, we want to combine multiple enum values. We can do this using the [Flags]
attribute:
[Flags]
public enum WeaponAttributes
{
None = 0,
Silenced = 1,
Scoped = 2,
ExtendedMag = 4,
LaserSight = 8
}
public WeaponAttributes currentAttributes = WeaponAttributes.Silenced | WeaponAttributes.Scoped;
This allows a weapon to have multiple attributes simultaneously.
Enum Methods
We can also create extension methods for enums to add functionality:
public static class WeaponTypeExtensions
{
public static float GetDamage(this WeaponType weaponType)
{
switch (weaponType)
{
case WeaponType.Pistol: return 10f;
case WeaponType.Shotgun: return 25f;
case WeaponType.AssaultRifle: return 15f;
case WeaponType.SniperRifle: return 50f;
default: return 0f;
}
}
}
// Usage
WeaponType currentWeapon = WeaponType.Shotgun;
float damage = currentWeapon.GetDamage();
This allows us to associate data or behavior with enum values directly.
FAQs
Q: Can I assign specific values to enum members?
A: Yes, you can assign integer values to enum members. For example:
public enum Difficulty
{
Easy = 1,
Medium = 2,
Hard = 3
}
Q: How do I convert a string to an enum value?
A: You can use Enum.Parse
or Enum.TryParse
:
WeaponType weapon = (WeaponType)Enum.Parse(typeof(WeaponType), "Pistol");
Q: Can I use enums in Unity’s Inspector?
A: Yes, enums show up as dropdown menus in the Unity Inspector, making them very convenient for designers to work with.
Q: Are there performance considerations when using enums?
A: Enums are very lightweight and perform similarly to integers, so they’re generally very efficient to use.
Q: Can I add or remove enum values at runtime?
A: No, enum values are fixed at compile-time and cannot be modified during runtime.
Enums are a powerful tool in C# and Unity game development. They help us write cleaner, more readable code and can significantly improve the organization of our game logic. Whether you’re managing weapon types in an FPS, character states in a platformer, or quest statuses in an RPG, enums provide a structured and type-safe way to handle these scenarios.
Remember, good use of enums can make your code more self-documenting and easier for other developers (or future you) to understand. So next time you find yourself using magic numbers or strings to represent a fixed set of options, consider if an enum might be a better fit!