Learning C# Operators: Tools for Crafting Logic and Performance
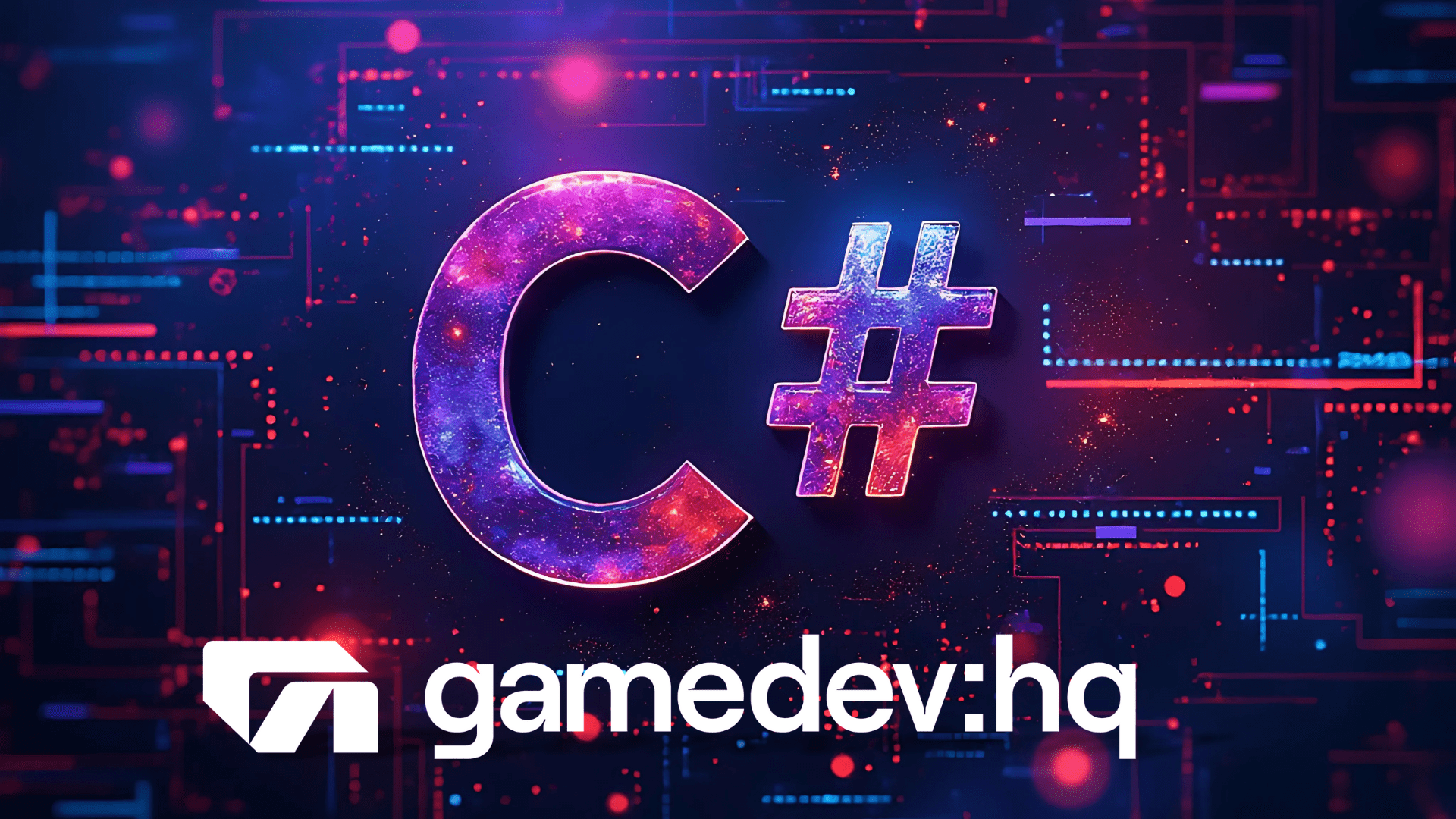
Think of C# operators as the gears in a well-oiled machine, each playing a crucial role in making your program function smoothly. Just as a mechanic uses different tools to fix cars, developers utilize a variety of operators to manipulate data and control the flow of their applications. For beginners, understanding these operators is essential to write clear and efficient code, whether you’re crafting simple applications or developing complex games.
Understanding C# Operators
C# operators are symbols that perform operations on one or more operands. They fall into several categories: arithmetic, assignment, comparison, logical, and bitwise operators, each serving a unique purpose in programming.
Sample Code Demonstrations of C# Operators
1. Arithmetic Operators
Arithmetic operators are used to perform basic mathematical operations such as addition, subtraction, multiplication, and division.
// Using arithmetic operators
int a = 10;
int b = 5;
int sum = a + b; // Addition
int diff = a - b; // Subtraction
int product = a * b; // Multiplication
int quotient = a / b; // Division
Console.WriteLine($"Sum: {sum}, Difference: {diff}, Product: {product}, Quotient: {quotient}");
In this example, we declare two integers and perform various arithmetic operations, demonstrating how these operators work.
2. Comparison Operators
Comparison operators are essential for decision-making in your code. They compare two values and return a boolean result.
// Using comparison operators
int x = 20;
int y = 15;
bool isGreater = x > y; // Greater than
bool isEqual = x == y; // Equal to
Console.WriteLine($"Is x greater than y? {isGreater}");
Console.WriteLine($"Is x equal to y? {isEqual}");
Here, we utilize comparison operators to determine if one number is greater than or equal to another, allowing us to make logical decisions.
3. Game Development: Logical Operators
In game development, logical operators are crucial for managing multiple conditions, such as player actions or game states.
// Using logical operators in a game scenario
bool hasKey = true;
bool doorIsLocked = true;
if (hasKey && doorIsLocked)
{
Console.WriteLine("Player can unlock the door.");
}
else
{
Console.WriteLine("Player cannot unlock the door.");
}
In this game scenario, logical operators help determine if a player can unlock a door based on certain conditions. By using the &&
operator, we ensure that both conditions must be true for the player to proceed.
C# operators are indispensable tools in a developer’s toolkit, much like the varied instruments used by a chef to prepare a meal. Each operator serves a different purpose, from performing basic calculations to making complex decisions in game logic. As you continue to explore the capabilities of C# operators, you’ll find that they are fundamental to crafting efficient, logical, and engaging programs. Whether you’re manipulating data or controlling game flow, mastering these operators will elevate your coding expertise.