Learn to Program with C# – CLASSES – Intermediate Unity Tutorial
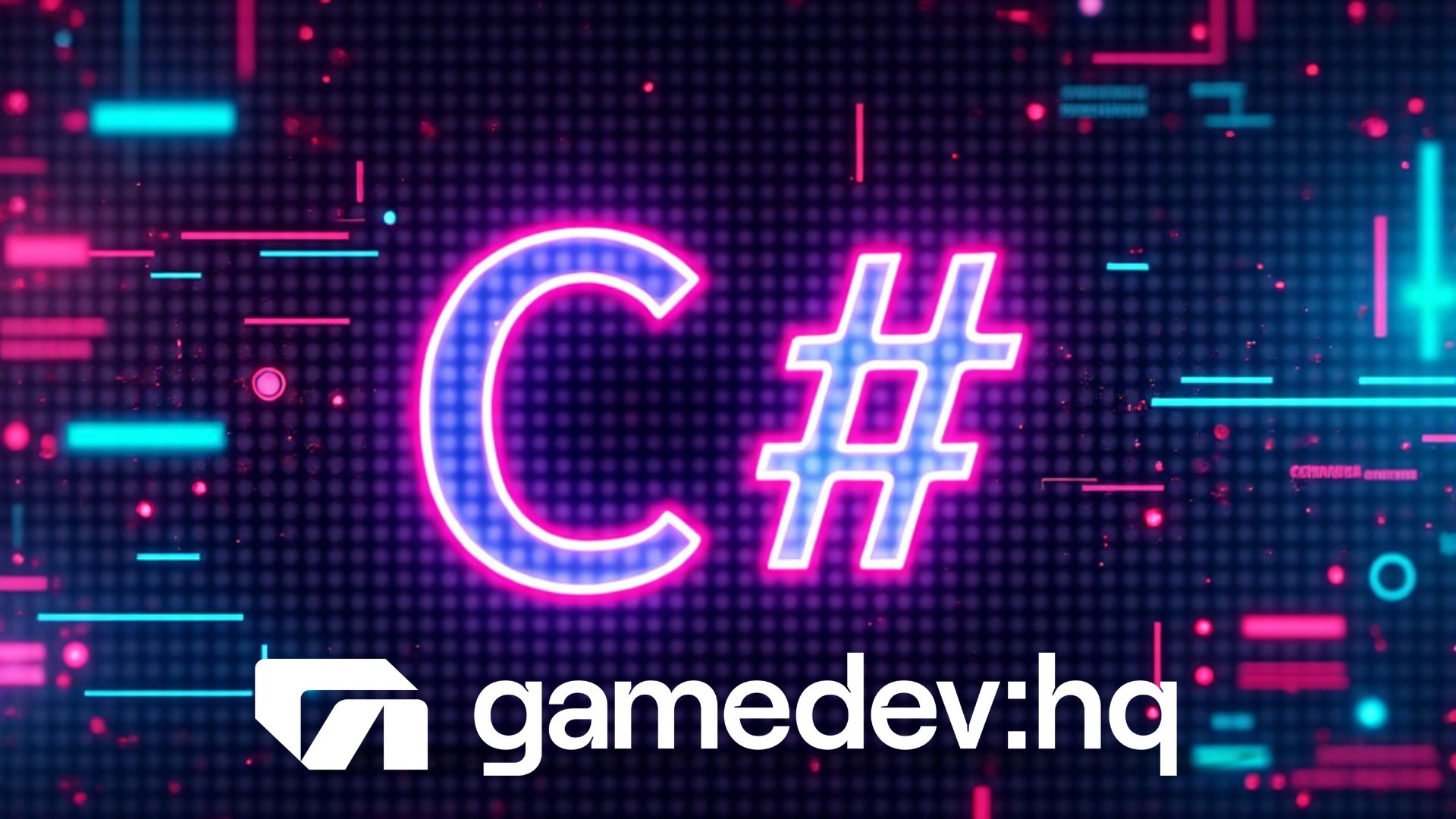
Let’s hop into the world of classes in C# and how they relate to Unity game development. Let’s explore this fundamental concept, which is crucial for creating structured and efficient code in your games.
Understanding Classes in C
Classes are the building blocks of object-oriented programming. Think of them as blueprints for creating objects in your game. Just as you might have a blueprint for a character in a platformer, a class defines the structure and behavior of objects in your code.
What is a Class?
A class is a user-defined type that encapsulates data and behavior. It’s like creating a custom game entity with its own properties and actions. For example, in a first-person shooter (FPS), you might have a Weapon class that defines attributes like damage, ammo capacity, and fire rate.
public class Weapon
{
public int damage;
public int ammoCapacity;
public float fireRate;
}
Namespaces and Using Statements
When working with classes, you’ll often encounter namespaces and using statements. These are like organizing your game assets into different folders for easy access.
Namespaces
Namespaces help organize and group related classes. They’re like different levels or areas in your game, each containing specific elements.
Using Statements
Using statements are shortcuts to access classes from other namespaces. It’s like having quick-access buttons to different parts of your game’s inventory.
using UnityEngine; // Gives access to Unity's built-in classes
using System.Collections; // Provides access to C# collections
Inheritance and MonoBehaviour
In Unity, most of your scripts will inherit from MonoBehaviour. This is like giving your game objects superpowers, allowing them to interact with Unity’s game loop.
public class PlayerController : MonoBehaviour
{
void Start() { /* Initialize player */ }
void Update() { /* Handle player input and movement */ }
}
Custom Classes vs. MonoBehaviour
Not all classes need to inherit from MonoBehaviour. Custom classes are useful for creating data structures or utility classes that don’t need to be attached to game objects.
Example: Item Class for an RPG
[System.Serializable]
public class Item
{
public int itemID;
public string itemName;
public string itemDescription;
}
This Item class could be used to define various items in your RPG without needing to create separate MonoBehaviour scripts for each item.
Using Custom Classes in Unity
To use custom classes in Unity, you often need to make them serializable. This allows Unity to display and modify these classes in the Inspector.
public class Inventory : MonoBehaviour
{
public Item[] items; // Array of custom Item class
}
Multiple Classes in One File
You can define multiple classes in a single file, which can be useful for organizing related classes:
public class Weapon { /* Weapon properties */ }
public class Armor { /* Armor properties */ }
public class Consumable { /* Consumable properties */ }
FAQs
Q: When should I use a custom class instead of inheriting from MonoBehaviour?
A: Use custom classes for data structures, utility functions, or any logic that doesn’t need to be directly attached to a GameObject in Unity.
Q: How do I make my custom classes visible in the Unity Inspector?
A: Add the [System.Serializable] attribute to your custom class definition.
Q: Can I use Unity-specific types in my custom classes?
A: Yes, as long as you include the ‘using UnityEngine;’ statement at the top of your script.
Conclusion
Classes are essential in game development with C# and Unity. They allow you to create structured, reusable code that can represent anything from game items to complex game systems. As you continue to develop your games, you’ll find classes to be invaluable tools in your programming toolkit.
Remember, just as you carefully design levels and characters in your game, take the time to design your classes thoughtfully. Well-structured classes can make your game development process smoother and more efficient, allowing you to focus on creating amazing gameplay experiences.