Learn to Program with C#: Interfaces – Advanced Unity Tutorial
I've found interfaces to be incredibly useful, especially when creating complex systems in games.
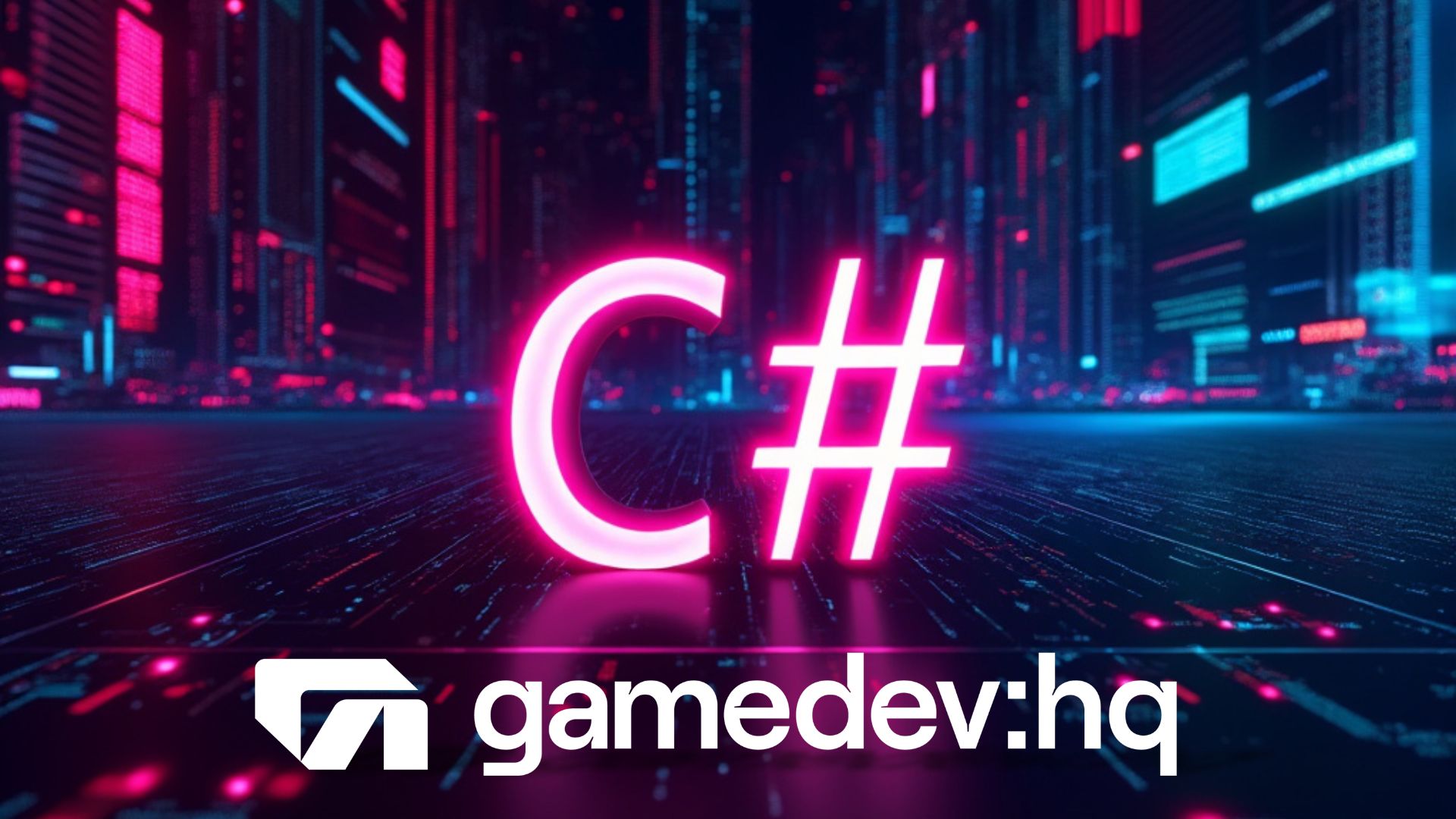
Hey there! I’m here to guide you through the world of interfaces in C# and Unity. As a game developer, I’ve found interfaces to be incredibly useful, especially when creating complex systems in games. Let’s dive into this topic, and I’ll use some game development analogies to help explain the concepts.
What Are Interfaces?
Interfaces in C# are like the rulebook for a board game. They define a set of rules (methods and properties) that any class implementing the interface must follow. Just as every player in a board game must follow the same rules, every class that implements an interface must include all the methods and properties defined in that interface.
In the context of game development, think of interfaces as a way to ensure that different game objects can interact with each other in a consistent way, even if their internal workings are different.
Why Use Interfaces?
Interfaces provide several benefits in game development:
- Modularity: They allow you to create plug-and-play components for your game objects.
- Code Reusability: You can apply the same interface to multiple classes, promoting code reuse.
- Flexibility: Different classes can implement the same interface in unique ways, allowing for varied behavior.
For example, in a role-playing game (RPG), you might have an IDamageable
interface that both player characters and enemies implement. This ensures that any object that can take damage in your game world follows a consistent pattern, even if the internal damage calculations differ.
Creating an Interface
Let’s create our first interface. In Unity, create a new C# script and name it “Interfaces”. Open it in your code editor and replace the contents with:
using UnityEngine;
public interface IDamageable<T>
{
void Damage(T damageAmount);
}
public interface IHealable
{
int Health { get; set; }
}
Here, we’ve created two interfaces:
IDamageable<T>
: A generic interface for objects that can take damage.IHealable
: An interface for objects that have health and can be healed.
Implementing Interfaces
Now, let’s create a player class that implements these interfaces:
using UnityEngine;
public class Player : MonoBehaviour, IDamageable<float>, IHealable
{
public int Health { get; set; }
public void Damage(float damageAmount)
{
Health -= Mathf.RoundToInt(damageAmount);
Debug.Log($"Player took {damageAmount} damage. Current health: {Health}");
}
void Start()
{
Health = 100;
}
}
In this example, our Player
class implements both the IDamageable<float>
and IHealable
interfaces. It’s like giving our player character the ability to take damage and be healed in our RPG.
Using Generic Interfaces
Notice how we used IDamageable<float>
for the player? This allows us to use floating-point values for player damage, which might be useful for things like damage-over-time effects or critical hits.
Let’s create an enemy class that uses integer damage instead:
using UnityEngine;
public class Enemy : MonoBehaviour, IDamageable<int>, IHealable
{
public int Health { get; set; }
public void Damage(int damageAmount)
{
Health -= damageAmount;
Debug.Log($"Enemy took {damageAmount} damage. Current health: {Health}");
}
void Start()
{
Health = 50;
}
}
By using generic interfaces, we can have different damage types for different game objects, while still ensuring they all follow the same basic structure.
Benefits of Using Interfaces in Game Development
- Consistent Interaction: In our RPG example, any object that implements
IDamageable
can be damaged, regardless of whether it’s a player, enemy, or destructible object in the environment. - Easy Extension: If we later decide to add new damageable objects (like breakable crates), we can simply implement the
IDamageable
interface in their class. - Simplified Systems: We can create damage-dealing systems that work with any
IDamageable
object, without needing to know the specifics of how each object handles damage.
Advanced Use: Interface Segregation
As your game grows more complex, you might want to break down interfaces into smaller, more specific ones. This is known as the Interface Segregation Principle. For example, instead of a single ICharacter
interface, you might have:
public interface IMovable
{
void Move(Vector3 direction);
}
public interface IAttacker
{
void Attack(IDamageable<int> target);
}
public interface IInventory
{
void AddItem(Item item);
void RemoveItem(Item item);
}
Then, different game objects can implement only the interfaces they need:
public class Player : MonoBehaviour, IMovable, IAttacker, IInventory, IDamageable<float>, IHealable
{
// Implementation here
}
public class Enemy : MonoBehaviour, IMovable, IAttacker, IDamageable<int>, IHealable
{
// Implementation here
}
public class Chest : MonoBehaviour, IInventory, IDamageable<int>
{
// Implementation here
}
This approach allows for more flexible and maintainable code as your game grows in complexity.
FAQs
Q: Can interfaces contain variables?
A: No, interfaces cannot contain variables. They can only declare properties, methods, and events.
Q: Can a class implement multiple interfaces?
A: Yes, a class can implement multiple interfaces. This is one of the key advantages of interfaces over inheritance in C#.
Q: Are interface members public by default?
A: Yes, all interface members are implicitly public. You don’t need to (and shouldn’t) use the public
keyword when declaring interface members.
Q: Can interfaces inherit from other interfaces?
A: Yes, interfaces can inherit from other interfaces. This is called interface inheritance.
Q: How do interfaces differ from abstract classes?
A: While both define a contract, abstract classes can provide implementation details and have constructors. Interfaces are purely abstract and cannot contain any implementation.
Conclusion
Interfaces are a powerful tool in C# and Unity game development. They allow us to create modular, reusable, and flexible code. By defining contracts for our game objects, we ensure consistent behavior across different types of entities in our game world.
Remember, like crafting the perfect game mechanics, mastering interfaces takes practice. Start by identifying common behaviors in your game objects and create interfaces for them. As you become more comfortable, you’ll find interfaces becoming an indispensable part of your game development toolkit.
Now go forth and create amazing, well-structured games with the power of interfaces!