Learn to Program with C# – LOOPS (for, foreach, do, while) – Unity Beginner Tutorial
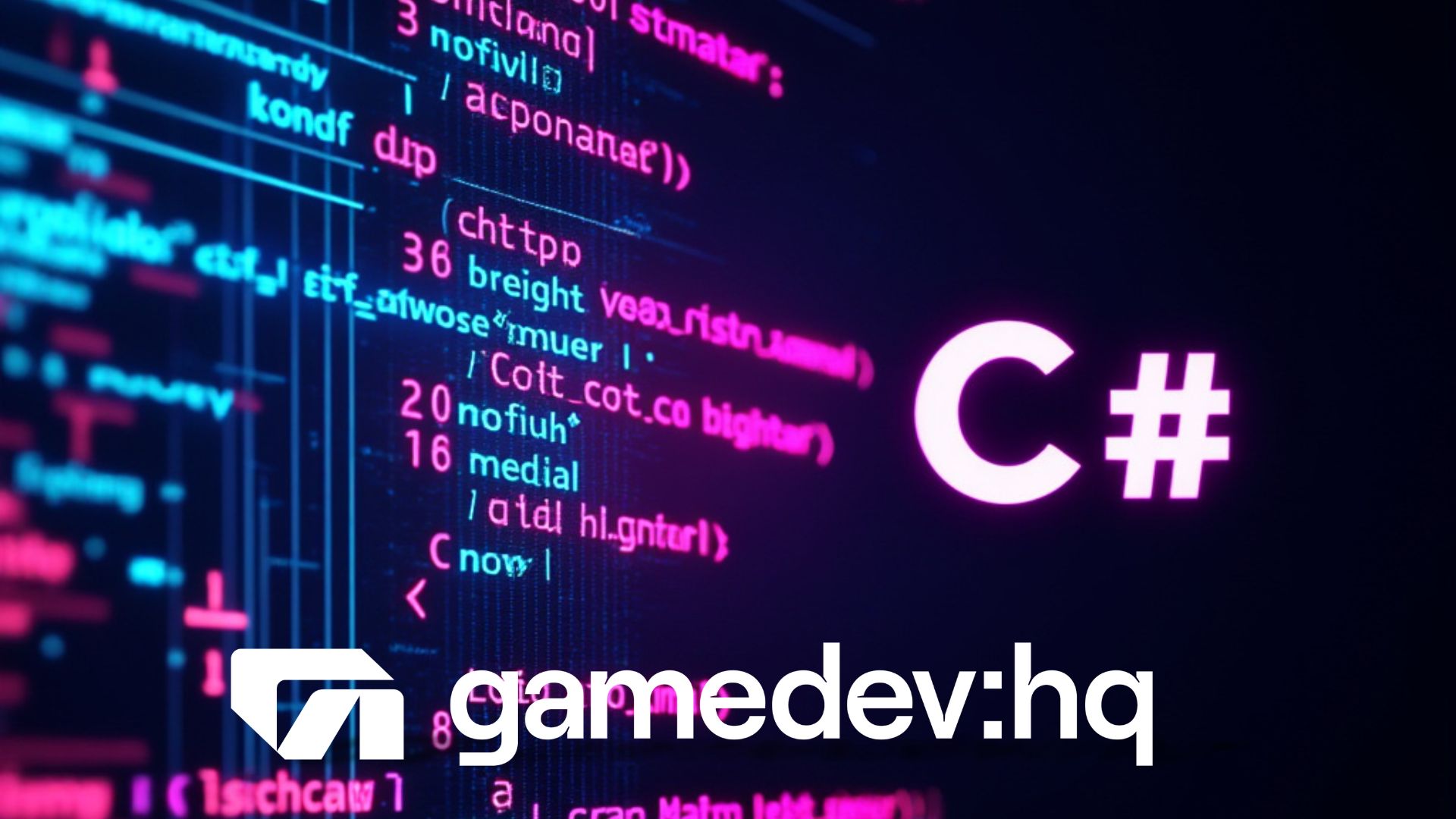
Ah, loops! The heartbeat of game development. Let me take you on a journey through the world of C# loops, as essential to programming as power-ups are to a platformer. Buckle up, because we’re about to loop-de-loop our way to coding mastery!
The Loop-de-Loop of Programming
Imagine you’re designing a side-scrolling platformer. Your hero needs to collect 100 coins scattered across the level. Would you write the same line of code 100 times to check for each coin? Of course not! That’s where loops come in, saving us from carpal tunnel and making our code as sleek as a well-oiled game engine.
In C#, we have four types of loops at our disposal: for, foreach, while, and do-while. Each has its own superpower, like different characters in an RPG. Let’s break them down one by one.
The “For” Loop: Your Trusty Sidekick
The “for” loop is like the reliable sidekick in your platformer – always there when you need to do something a specific number of times. Here’s how it looks:
for (int i = 0; i < 100; i++)
{
Debug.Log("Collecting coin number: " + i);
}
This loop will run 100 times, perfect for our coin-collecting scenario. It’s like telling your game, “For each coin from 0 to 99, do this.” The “i” variable keeps track of which iteration we’re on, like a coin counter in our game.
The “Foreach” Loop: The Smart Bomb
The “foreach” loop is C#’s smart bomb. It’s designed to work with collections (like arrays or lists) and is incredibly readable. Imagine you have an array of power-ups:
string[] powerUps = { "Speed Boost", "Invincibility", "Double Jump" };
foreach (string powerUp in powerups)
{
Debug.Log("Activating power-up: " + powerUp);
}
This loop will go through each power-up in our array, activating them one by one. It’s like your character automatically using every power-up they collect without you having to specify how many there are.
The “While” Loop: The Boss Battle
The “while” loop is like the boss battle of loops. It keeps going as long as a condition is true. Be careful, though – if the condition never becomes false, you’ll be stuck in an infinite loop, like a game that never ends!
int health = 100;
while (health > 0)
{
Debug.Log("Still fighting! Health: " + health);
health -= 10; // The boss hits us for 10 damage each turn
}
This loop continues as long as our health is above 0. It’s perfect for situations where you don’t know exactly how many times something needs to happen, like battling a boss with an unknown amount of health.
The “Do-While” Loop: The Tutorial Level
The “do-while” loop is like the tutorial level of your game. It always runs at least once, then checks if it should continue. It’s great for situations where you want to ensure something happens at least once:
int attempts = 0;
do
{
attempts++;
Debug.Log("Attempt " + attempts + " to defeat the boss");
} while (attempts < 3);
This loop will always give us at least one attempt at the boss, then continue up to three attempts total. It’s like giving the player a guaranteed first try before checking if they want to continue.
Practical Applications in Game Development
Now, let’s see how these loops might be used in real game development scenarios:
- Spawning enemies:
for (int i = 0; i < 10; i++)
{
SpawnEnemy();
}
- Updating all game objects:
foreach (GameObject obj in gameObjects)
{
obj.Update();
}
- Game over check:
while (playerIsAlive)
{
PlayGame();
}
- Player input:
do
{
GetPlayerInput();
} while (!inputIsValid);
FAQs
Q: When should I use a “for” loop versus a “foreach” loop?
A: Use a “for” loop when you need to iterate a specific number of times or need access to the index. Use “foreach” when working with collections and you don’t need the index.
Q: Can I nest loops inside each other?
A: Absolutely! Nested loops are common in game development, like when you’re working with 2D grids or matrices.
Q: How do I break out of a loop early?
A: Use the “break” keyword to exit a loop prematurely. This is useful when you’ve found what you’re looking for and don’t need to continue looping.
Q: What’s the difference between “i++” and “++i” in a for loop?
A: In most cases, they work the same in a for loop. “i++” (postfix) increments after the current line, while “++i” (prefix) increments before. In a for loop, this distinction doesn’t usually matter.
Loops are the backbone of efficient programming, allowing us to repeat actions without repeating code. They’re as crucial to game development as checkpoints are to a challenging platformer. Whether you’re spawning enemies, updating game objects, or processing player input, mastering loops will level up your coding skills significantly.
Remember, practice makes perfect. Try implementing these loops in your own projects, and soon you’ll be looping through code as effortlessly as a speedrunner blazing through levels. Happy coding, and may your games be forever loop-optimized!