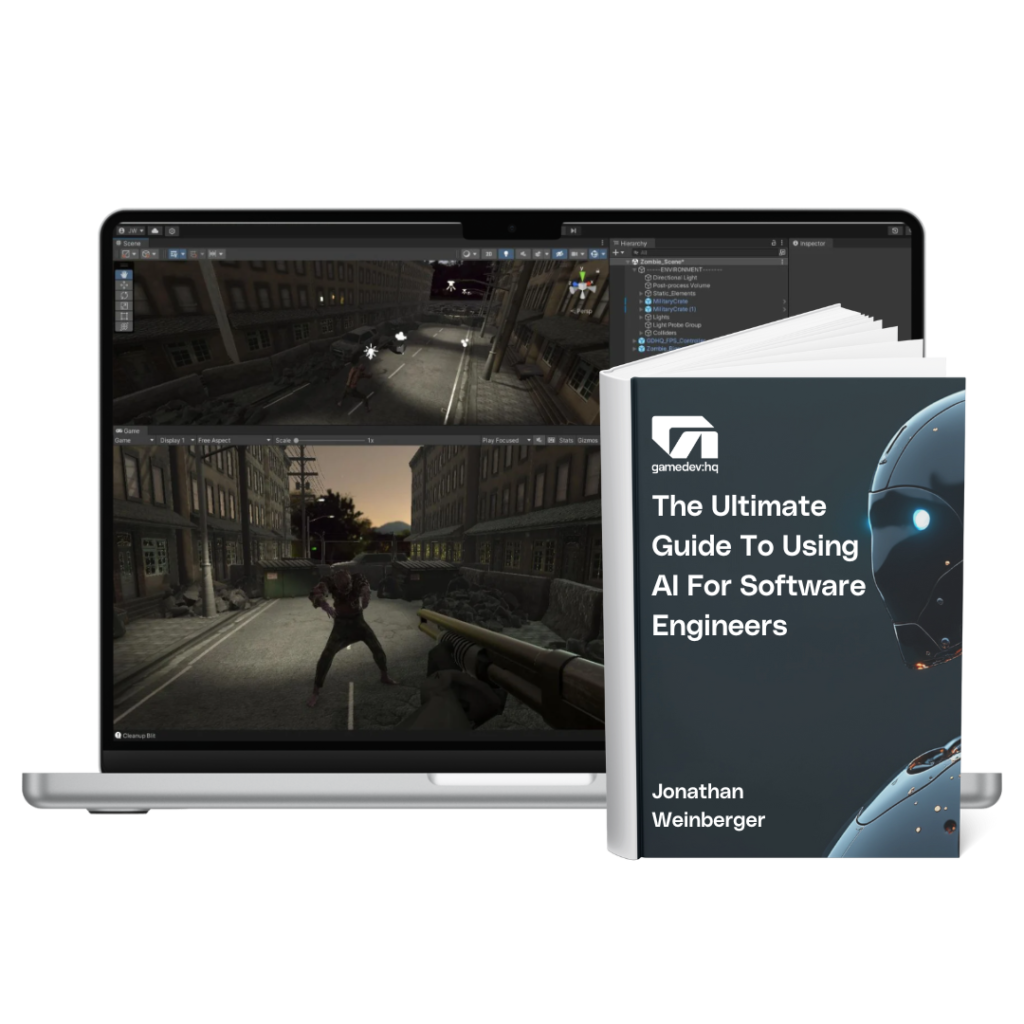
Learn to Program with C# – PROPERTIES – Intermediate Unity Tutorial
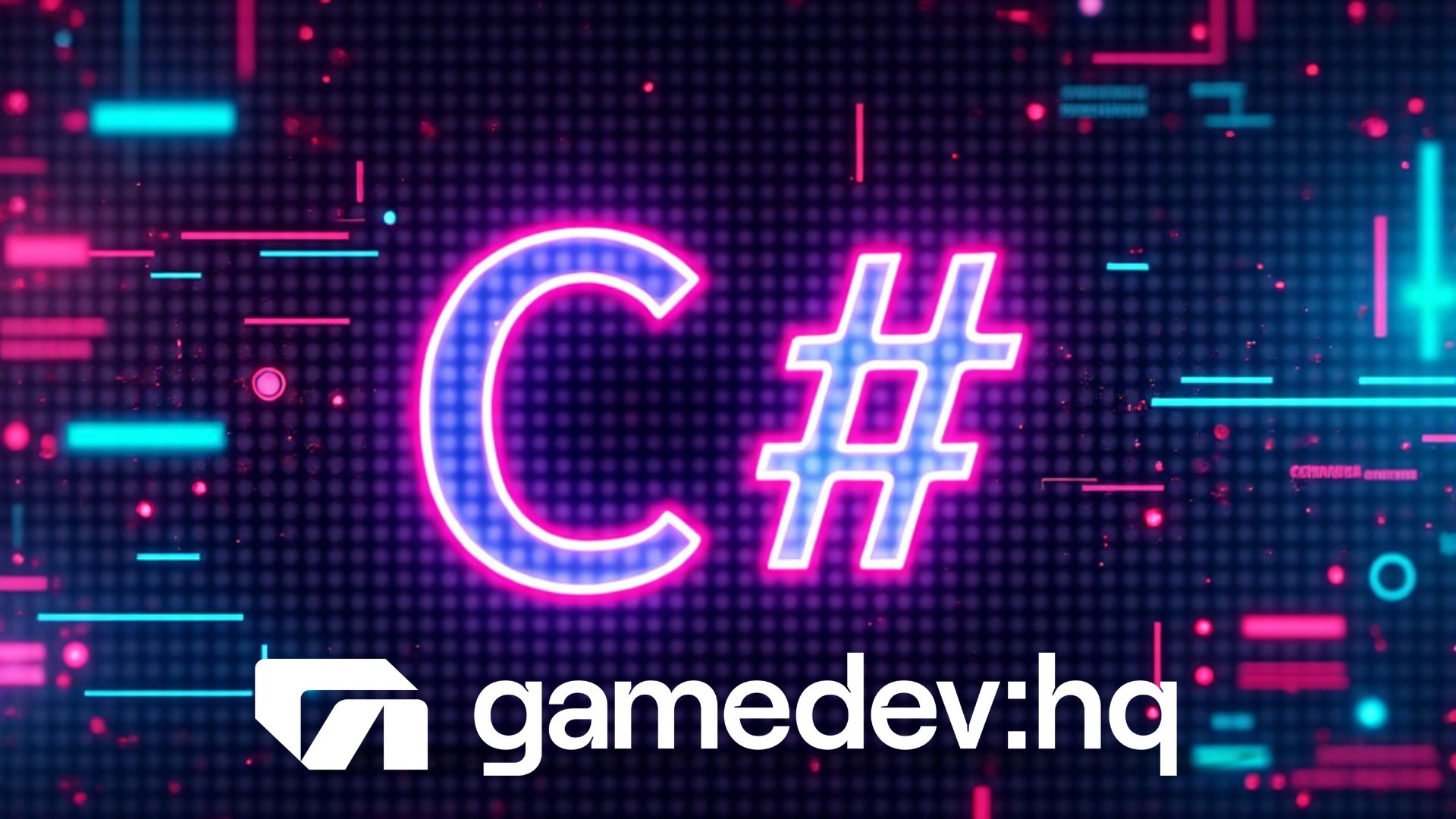
I’m about to take you on a thrilling journey through the world of properties in C#, as exciting as unlocking new abilities in a role-playing game (RPG). Let’s dive into this crucial aspect of programming that can level up your coding skills!
What Are Properties?
Properties in C# are like the stats of your RPG character. They’re special class members that allow you to read, write, or compute the value of a private field. Think of them as the gatekeepers of your data, controlling how it’s accessed and modified.
In the realm of Unity game development, you’ve likely encountered properties without even realizing it. For instance, when you use Time.deltaTime
or Time.timeScale
, you’re interacting with properties. These are not just simple variables; they’re sophisticated constructs that offer more control and flexibility.
The Anatomy of a Property
A property consists of two main parts:
- Getter: This is like your character’s perception skill. It allows you to retrieve the value of a private field.
- Setter: Think of this as your character’s ability to equip items. It lets you assign a value to a private field.
Here’s a basic example of how a property looks:
private int _myAge;
public int MyAge
{
get { return _myAge; }
set { _myAge = value; }
}
In this code, _myAge
is our private field (like a hidden stat), and MyAge
is the property that provides controlled access to it.
Why Use Properties?
Properties are like the enchanted equipment in your RPG inventory. They offer several advantages:
- Encapsulation: They hide the internal representation of data, just like how your character’s armor hides their vulnerable spots.
- Validation: You can add logic to validate data before setting it, similar to checking if your character meets the level requirement for a powerful weapon.
- Computed Values: Properties can return calculated values, like how your character’s total attack power might be a combination of base stats and equipment bonuses.
- Flexibility: You can change the internal implementation without affecting the public interface, much like swapping out your character’s gear without changing their overall appearance.
Types of Properties
1. Read-Only Properties
These are like your character’s base stats that can’t be changed directly:
public int Level
{
get { return _level; }
}
2. Write-Only Properties
Rare, but useful in certain scenarios, like setting a password:
public string Password
{
set { _password = value; }
}
3. Read-Write Properties
The most common type, allowing both reading and writing:
public int Health
{
get { return _health; }
set { _health = value; }
}
4. Auto-Implemented Properties
These are like the quick-equip slots in your RPG inventory. They’re concise and useful when you don’t need custom logic:
public int Mana { get; set; }
Using Properties in Unity
In Unity game development, properties are incredibly useful for creating clean, maintainable code. Let’s say we’re creating a player character for our RPG:
public class PlayerCharacter : MonoBehaviour
{
private int _health;
public int Health
{
get { return _health; }
set
{
_health = Mathf.Clamp(value, 0, 100);
if (_health <= 0)
GameOver();
}
}
private void GameOver()
{
Debug.Log("Game Over!");
}
}
In this example, the Health
property ensures that the player’s health stays between 0 and 100, and triggers a game over when it reaches zero. This is much more powerful than a simple public variable!
Advanced Property Techniques
1. Expression-Bodied Properties
For simple properties, you can use a more concise syntax:
public string FullName => $"{FirstName} {LastName}";
2. Computed Properties
These calculate a value based on other properties:
public float AttackPower => BaseAttack * (1 + StrengthBonus / 100f);
3. Properties with Different Accessibility Levels
You can have different access levels for getters and setters:
public int Experience
{
get { return _experience; }
private set { _experience = value; }
}
This allows anyone to read the experience, but only the class itself can modify it.
Best Practices
- Use properties instead of public fields for better encapsulation.
- Keep property getters and setters simple. If complex logic is needed, consider using methods instead.
- Use auto-implemented properties when no additional logic is required.
- Consider making setters private or protected if they shouldn’t be modified externally.
FAQs
Q: When should I use properties instead of public fields?
A: Use properties when you need control over how a value is accessed or modified, or when you want to compute a value dynamically.
Q: Can properties have side effects?
A: Yes, but use this sparingly. Side effects in getters can lead to unexpected behavior.
Q: Are properties slower than fields?
A: For simple properties, the performance difference is negligible. The benefits of encapsulation usually outweigh any minor performance costs.
Q: Can I use properties in interfaces?
A: Absolutely! Interfaces can define properties, which implementing classes must provide.
Properties in C# are a powerful tool in your programming arsenal. They’re like the magical abilities of your RPG character, allowing you to control and enhance your code in ways that simple variables can’t match. By mastering properties, you’re leveling up your C# skills and preparing yourself for more advanced game development challenges. So go forth, brave coder, and may your properties serve you well in your quest for programming excellence!