C# Regex: Powerful Pattern Matching for Game Developers
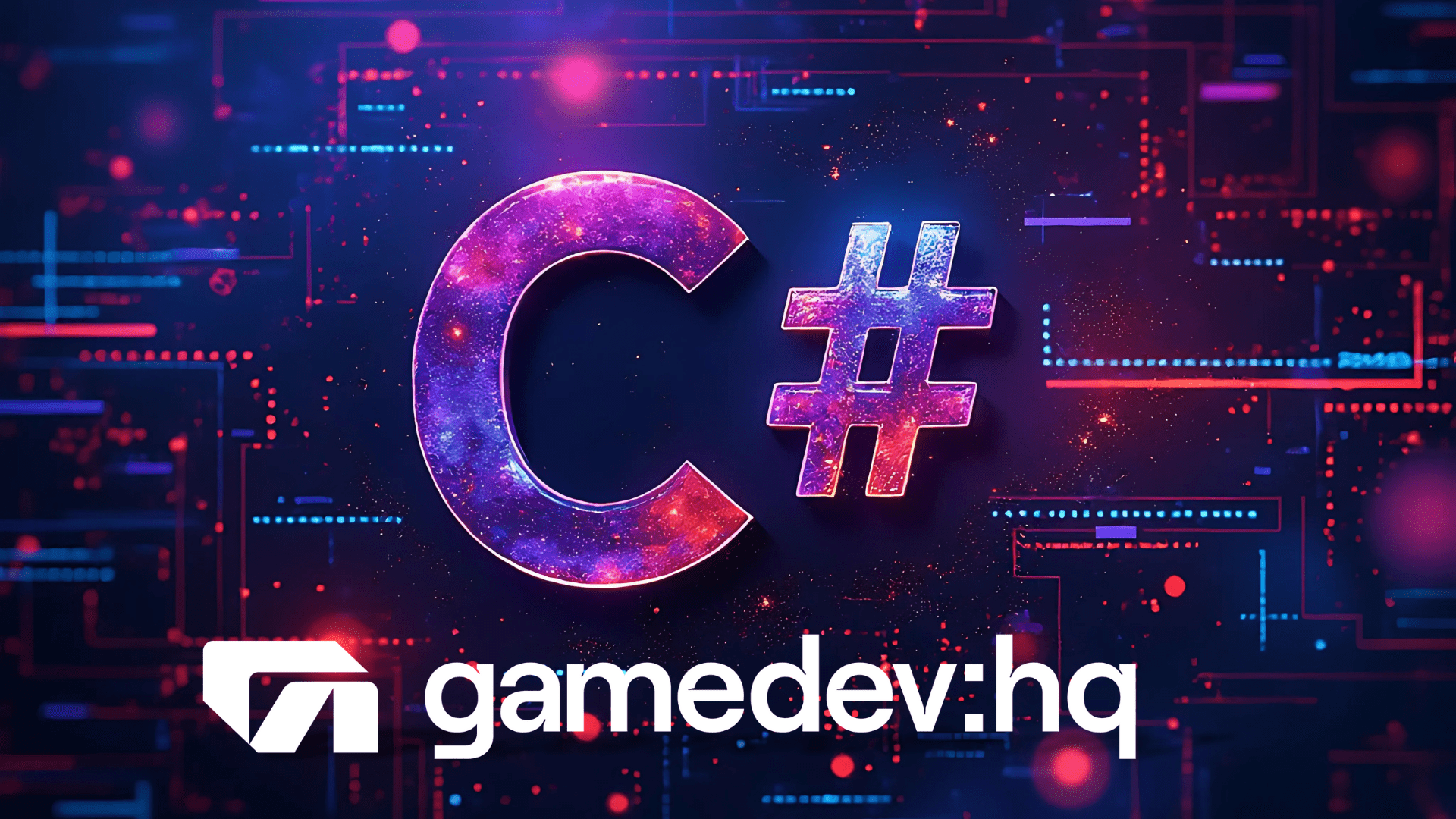
C Sharp Regular Expression: Powerful Pattern Matching
As a game developer, I’ve found C# regex to be an incredibly useful tool, especially when working on text-based adventures or dialogue systems. Let me walk you through the basics and some advanced techniques of using regular expressions in C#.
What is Regex?
Regex, short for regular expressions, is a sequence of characters that defines a search pattern. It’s like creating a cheat code for finding specific text patterns. In C#, we use the System.Text.RegularExpressions namespace to work with regex.
Think of regex as a super-powered “Find” function in your favorite text editor. Instead of just searching for exact matches, you can search for patterns. It’s like setting up intricate traps in a stealth game to catch specific types of enemies.
Basic Regex in C
Let’s start with a simple example:
using System;
using System.Text.RegularExpressions;
string text = "The player has 100 health and 50 mana.";
string pattern = @"\d+";
MatchCollection matches = Regex.Matches(text, pattern);
foreach (Match match in matches)
{
Console.WriteLine(match.Value);
}
This code will output:
100
50
Here, \d+
is our regex pattern. It matches one or more digits. The @
before the string makes it a verbatim string literal, which is useful for regex as we don’t need to escape backslashes.
Common Regex Patterns
^
– Start of a line$
– End of a line.
– Any character except newline*
– Zero or more occurrences+
– One or more occurrences?
– Zero or one occurrence\w
– Word character (letter, digit, or underscore)\s
– Whitespace character
These patterns are like the basic moves in a fighting game. Combining them creates powerful combos.
Regex Groups
Groups in regex are like collectibles in a platformer. They allow you to capture specific parts of a match. Here’s an example:
string text = "Player1 scored 1000 points. Player2 scored 1500 points.";
string pattern = @"(\w+) scored (\d+) points";
MatchCollection matches = Regex.Matches(text, pattern);
foreach (Match match in matches)
{
Console.WriteLine($"{match.Groups[1].Value} scored {match.Groups[2].Value} points");
}
This will output:
Player1 scored 1000 points
Player2 scored 1500 points
Regex Replace
Regex replace is like a spell that transforms text. It’s incredibly useful for modifying strings based on patterns:
string text = "The sword deals 10-20 damage.";
string pattern = @"\d+";
string replacement = "X";
string result = Regex.Replace(text, pattern, replacement);
Console.WriteLine(result);
Output:
The sword deals X-X damage.
Regex Options
C# regex supports various options to modify how the pattern matching works. These are like difficulty settings in a game:
- IgnoreCase: Makes the regex case-insensitive
- Multiline: Changes how ^ and $ work
- Singleline: Makes . match newlines
Example:
string text = "PLAYER health: 100\nENEMY health: 50";
string pattern = @"^player";
RegexOptions options = RegexOptions.IgnoreCase | RegexOptions.Multiline;
MatchCollection matches = Regex.Matches(text, pattern, options);
Console.WriteLine(matches.Count); // Outputs: 1
Performance Considerations
When using regex in game development, especially in real-time scenarios, performance is crucial. Here are some tips:
- Use compiled regex for patterns you’ll use multiple times:
Regex compiledRegex = new Regex(@"\d+", RegexOptions.Compiled);
- Use non-backtracking regex (available in .NET 7+) for better performance:
Regex nonBacktrackingRegex = new Regex(@"\d+", RegexOptions.NonBacktracking);
- Avoid overuse of backtracking. It’s like having too many nested loops in your game logic – it can slow things down.
Practical Examples in Game Development
- Parsing command inputs:
string input = "move north 5 steps";
string pattern = @"^(\w+)\s+(\w+)\s+(\d+)\s+(\w+)$";
Match match = Regex.Match(input, pattern);
if (match.Success)
{
string action = match.Groups[1].Value;
string direction = match.Groups[2].Value;
int steps = int.Parse(match.Groups[3].Value);
Console.WriteLine($"Action: {action}, Direction: {direction}, Steps: {steps}");
}
- Validating player names:
string playerName = "Player123";
string pattern = @"^[a-zA-Z][a-zA-Z0-9]{2,15}$";
if (Regex.IsMatch(playerName, pattern))
{
Console.WriteLine("Valid player name");
}
else
{
Console.WriteLine("Invalid player name");
}
- Parsing game logs:
string log = "[2024-10-15 14:30:22] Player 'Hero1' defeated 'Dragon' (XP +500)";
string pattern = @"\[(\d{4}-\d{2}-\d{2} \d{2}:\d{2}:\d{2})\] Player '(\w+)' defeated '(\w+)' \(XP \+(\d+)\)";
Match match = Regex.Match(log, pattern);
if (match.Success)
{
DateTime timestamp = DateTime.Parse(match.Groups[1].Value);
string player = match.Groups[2].Value;
string enemy = match.Groups[3].Value;
int xp = int.Parse(match.Groups[4].Value);
Console.WriteLine($"At {timestamp}, {player} defeated {enemy} and gained {xp} XP");
}
Common Pitfalls and How to Avoid Them
- Greedy vs. Lazy Quantifiers:
By default, quantifiers like * and + are greedy, meaning they match as much as possible. This can lead to unexpected results. Use lazy quantifiers (*? and +?) when you want to match as little as possible. - Catastrophic Backtracking:
Some regex patterns can lead to exponential time complexity. For example, matching nested structures can be problematic. Use atomic groups (?>…) or possessive quantifiers (*+, ++) to prevent backtracking. - Overreliance on Regex:
While regex is powerful, it’s not always the best tool. For simple string operations, methods like String.Contains() or String.StartsWith() are often faster and more readable.
Conclusion
C# regex is a powerful tool in a game developer’s arsenal. It’s particularly useful for text-based games, parsing configuration files, or processing user inputs. Like mastering a complex game mechanic, becoming proficient with regex takes practice, but the payoff in terms of code efficiency and flexibility is substantial.
Remember, the key to using regex effectively is to start simple and build complexity as needed. Don’t try to create an epic boss-battle regex right from the start. Begin with small, manageable patterns and combine them as you gain confidence.
By incorporating regex into your C# game development toolkit, you’re equipping yourself with a versatile and powerful ability to manipulate and analyze text. Whether you’re creating dialogue systems, parsing game data, or validating user inputs, regex can significantly streamline your code and enhance your game’s functionality.