Learn to Program with C# – SWITCH STATEMENTS – Unity Tutorial
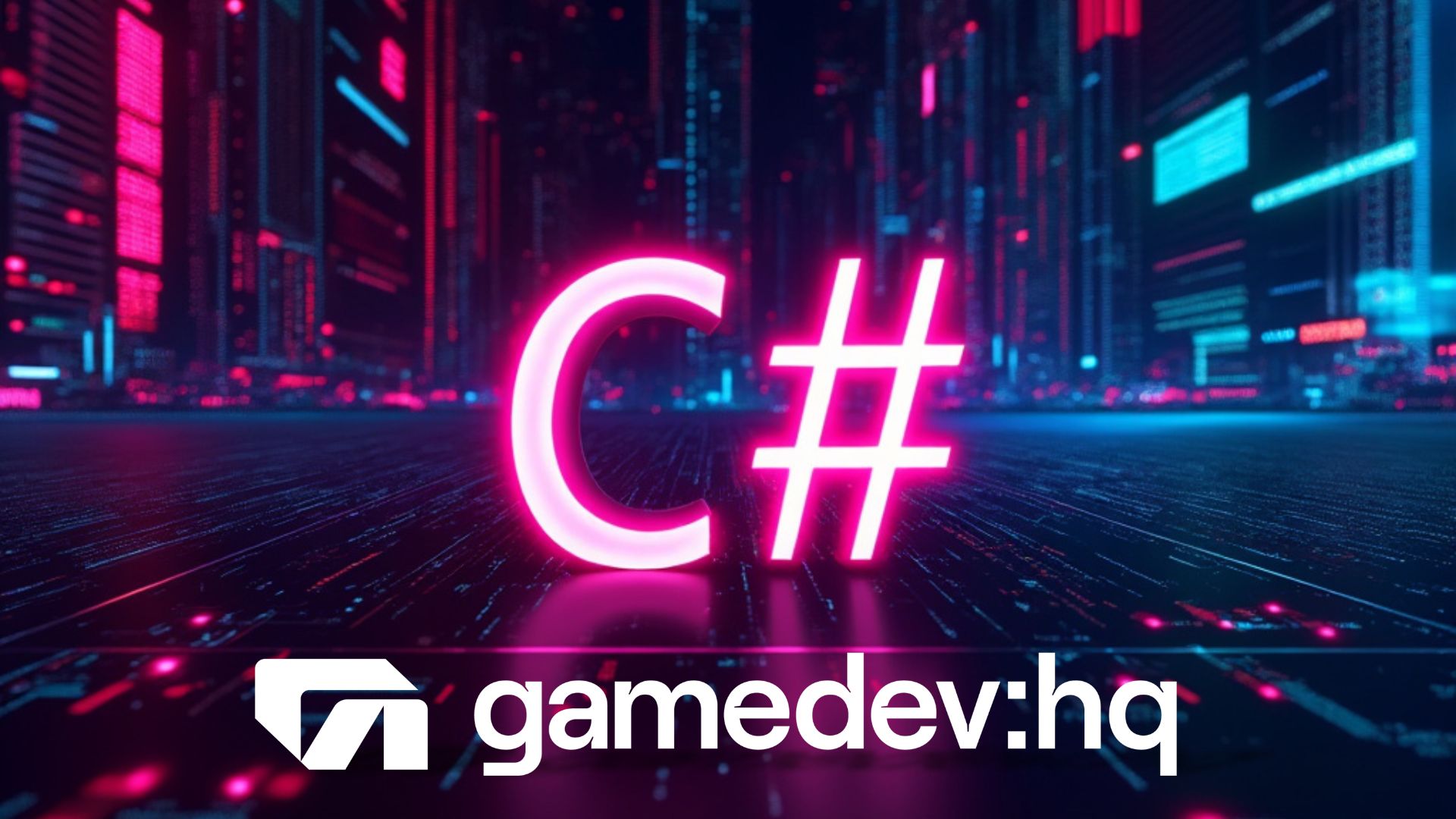
The switch statement – a programmer’s trusty sidekick in the epic quest of game development! Let me break this down for you in a way that’ll make even the most complex code feel like a walk in the park.
The Power of Switch Statements
Imagine you’re crafting a role-playing game (RPG) where players choose their character class. You could use a series of if-else statements to handle each selection, but that’s like forcing your hero to navigate a maze when there’s a perfectly good teleportation spell available. Enter the switch statement – your code’s magic portal to efficiency!
public class CharacterCreator : MonoBehaviour
{
public int selectedClass;
void Start()
{
switch (selectedClass)
{
case 0:
Debug.Log("You've chosen the mighty Warrior!");
break;
case 1:
Debug.Log("A cunning Rogue joins the party!");
break;
case 2:
Debug.Log("The wise Mage emerges from the tower!");
break;
default:
Debug.Log("Unknown class. Are you a secret unlockable character?");
break;
}
}
}
In this RPG scenario, the switch statement acts like a character selection screen. It’s clean, organized, and doesn’t keep the player (or the processor) waiting!
Switch vs If-Else: The Epic Showdown
While if-else statements are like choosing dialogue options in a conversation-heavy RPG, switch statements are more like a well-designed skill tree. They’re both useful, but switch statements shine when you’re dealing with multiple, specific conditions.
// If-Else approach (verbose and potentially messy)
if (playerLevel == 1)
UnlockBasicSkill();
else if (playerLevel == 5)
UnlockAdvancedSkill();
else if (playerLevel == 10)
UnlockExpertSkill();
else
Debug.Log("No new skills available.");
// Switch approach (clean and efficient)
switch (playerLevel)
{
case 1:
UnlockBasicSkill();
break;
case 5:
UnlockAdvancedSkill();
break;
case 10:
UnlockExpertSkill();
break;
default:
Debug.Log("No new skills available.");
break;
}
The Anatomy of a Switch Statement
Let’s dissect our switch statement like we’re examining a rare monster in our RPG bestiary:
- The switch keyword: This is the incantation that begins our magical selection process.
- The variable: In parentheses, we specify what we’re examining (like
playerLevel
orselectedClass
). - Case statements: Each
case
is like a possible outcome in our adventure. - Break statements: These are the exit portals from each case. Without them, your code might fall through to the next case!
- Default case: This is your “else” equivalent, handling any unexpected scenarios.
Switch Statement Best Practices
- Use for equality comparisons: Switch statements work best when you’re comparing a variable against specific values.
- Don’t forget the break: Omitting
break
can lead to unexpected behavior, like accidentally triggering multiple cases. - Consider readability: If you have only two or three simple conditions, an if-else might be more readable.
- Watch out for complex cases: If your cases require complex logic, consider using if-else instead.
FAQs
Q: Can I use switch statements with strings?
A: Absolutely! C# supports switch statements with strings, making them great for menu systems or command parsing.
Q: What happens if I forget a break statement?
A: The code will “fall through” to the next case, potentially causing unintended behavior. Always use break unless you specifically want this behavior.
Q: Can I use variables in case statements?
A: In C# 7.0 and later, you can use pattern matching in switch statements, allowing for more complex case conditions.
Q: Are switch statements faster than if-else chains?
A: For a small number of cases, the performance difference is negligible. For larger numbers, switch statements can be more efficient, especially if the compiler can optimize them into a jump table.
Leveling Up Your Switch Skills
As you progress in your coding journey, you’ll encounter more advanced uses of switch statements. Here are a few to look forward to:
- Pattern matching: Introduced in C# 7.0, this allows for more complex case conditions.
- Switch expressions: A more concise way to write switch statements, great for assigning values based on conditions.
- When clauses: These allow you to add additional conditions to your cases.
Remember, like mastering any skill in an RPG, becoming proficient with switch statements takes practice. Don’t be afraid to experiment and refactor your code to see where they fit best.
In conclusion, switch statements are a powerful tool in your C# arsenal. They can make your code cleaner, more efficient, and easier to read – especially when dealing with multiple specific conditions. So the next time you find yourself writing a long chain of if-else statements, consider if a switch statement might be the level-up your code needs!
Now go forth, brave coder, and may your switch statements always find their perfect case!