Learn to Program with C# – STRUCTS VS CLASSES – Advanced Unity Tutorial
I'm excited to dive into the world of structs and classes in C# for Unity development. Let's explore these concepts as if we're designing a first-person shooter (FPS) game.
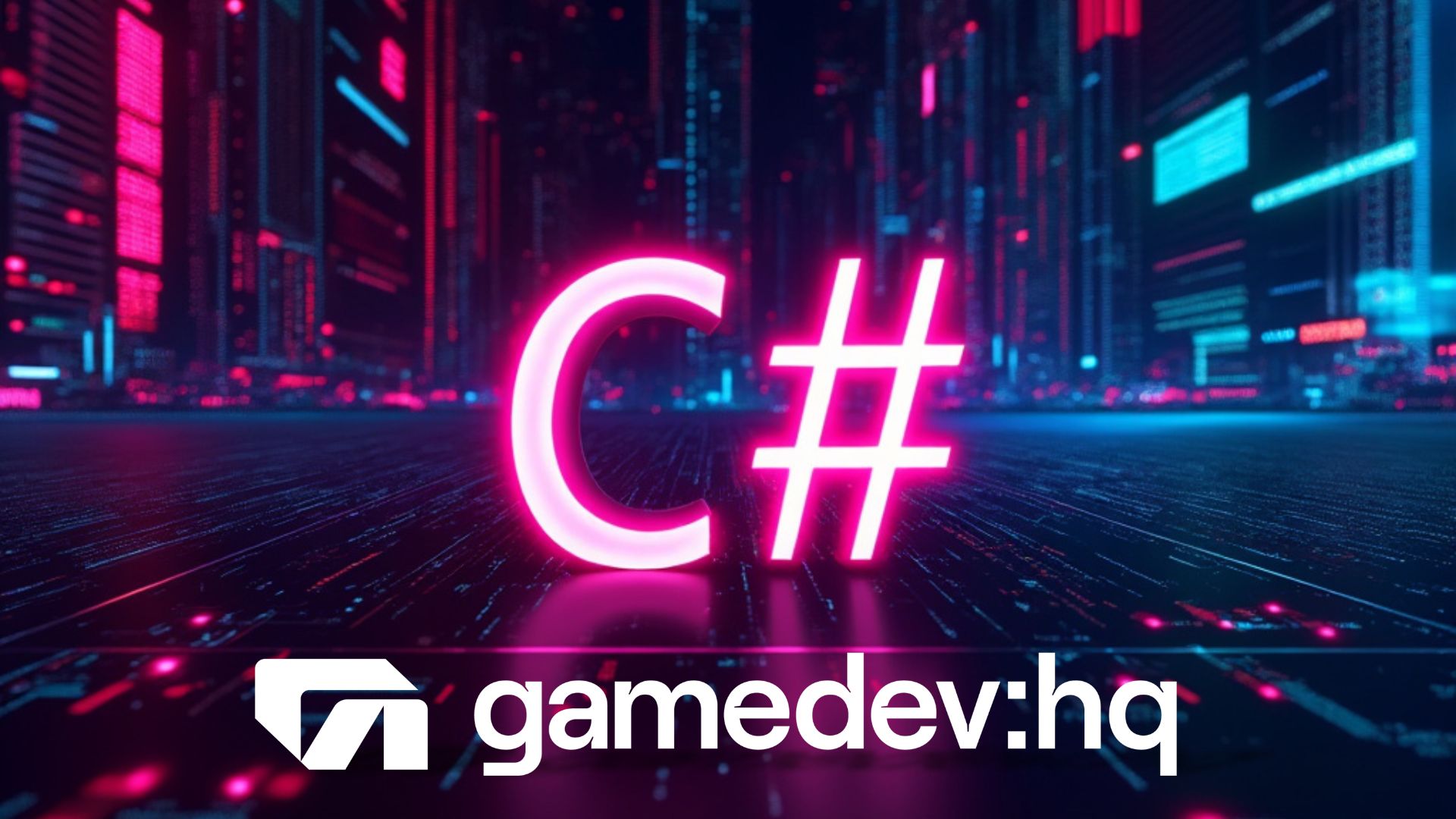
I’m excited to dive into the world of structs and classes in C# for Unity development. Let’s explore these concepts as if we’re designing a first-person shooter (FPS) game.
Understanding Structs and Classes
Structs and classes are both data structures in C# that allow us to group related variables and functions together. However, they have some key differences that affect how we use them in game development.
Similarities
Both structs and classes can have:
- Fields (variables)
- Methods
- Constructors
- Constants
- Properties
Key Differences
- Inheritance: Classes can inherit from other classes, while structs cannot inherit.
- Memory allocation: Classes are reference types (stored on the heap), while structs are value types (stored on the stack).
- Default constructor: Structs have a default parameterless constructor, while classes require explicit constructor declaration if needed.
When to Use Structs vs Classes
In our FPS game, we’ll use structs for small, lightweight data structures and classes for more complex objects.
Using Structs
Structs are ideal for small groups of related variables, typically with less than five members. In our FPS game, we might use structs for:
- Weapon Stats:
public struct WeaponStats
{
public int damage;
public float fireRate;
public int ammoCapacity;
}
- Player Position:
public struct Position
{
public float x;
public float y;
public float z;
}
Using Classes
Classes are better suited for more complex objects or when inheritance is needed. In our FPS game, we might use classes for:
- Player Class:
public class Player : MonoBehaviour
{
public int health;
public WeaponStats currentWeapon;
public Position position;
public void TakeDamage(int amount) { /* ... */ }
public void Shoot() { /* ... */ }
}
- Enemy Class Hierarchy:
public class Enemy : MonoBehaviour
{
public int health;
public virtual void Attack() { /* ... */ }
}
public class Zombie : Enemy
{
public override void Attack() { /* ... */ }
}
public class Robot : Enemy
{
public override void Attack() { /* ... */ }
}
Creating and Using Structs
Let’s create a struct for our weapon in the FPS game:
public struct Weapon
{
public int itemId;
public string itemName;
public int attackBonus;
public int agilityBonus;
}
// Creating a new weapon
Weapon assaultRifle;
assaultRifle.itemId = 1;
assaultRifle.itemName = "Assault Rifle";
assaultRifle.attackBonus = 15;
assaultRifle.agilityBonus = 5;
Notice that we don’t use the new
keyword when creating a struct instance.
Class Inheritance
In our FPS game, we can use class inheritance to create different types of weapons:
public class Item
{
public int itemId;
public string itemName;
}
public class Weapon : Item
{
public int attackBonus;
public int agilityBonus;
}
// Creating a new weapon
Weapon sniperRifle = new Weapon();
sniperRifle.itemId = 2;
sniperRifle.itemName = "Sniper Rifle";
sniperRifle.attackBonus = 30;
sniperRifle.agilityBonus = -5;
Performance Considerations
In game development, performance is crucial. Structs can be more efficient for small, frequently used data types because they’re stored on the stack. This can lead to faster access times and less garbage collection overhead.
For example, in our FPS game, using a struct for bullet data could be more efficient:
public struct Bullet
{
public Vector3 position;
public Vector3 velocity;
public float damage;
}
Using this struct instead of a class for bullets could improve performance, especially when dealing with hundreds or thousands of bullets in a scene.
Serialization
When working with Unity’s inspector, we need to consider serialization. Both structs and classes can be serialized, but we need to use the [System.Serializable]
attribute:
[System.Serializable]
public struct WeaponStats
{
public int damage;
public float fireRate;
public int ammoCapacity;
}
public class Player : MonoBehaviour
{
public WeaponStats primaryWeapon;
}
This allows us to see and edit the WeaponStats
in the Unity Inspector.
FAQs
Q: Can structs have methods?
A: Yes, structs can have methods, just like classes.
Q: When should I use a struct instead of a class?
A: Use structs for small, lightweight data structures with less than five members. Use classes for more complex objects or when you need inheritance.
Q: Can structs inherit from interfaces?
A: Yes, structs can implement interfaces, but they cannot inherit from other structs or classes.
Q: Are there performance benefits to using structs?
A: Structs can offer performance benefits for small, frequently used data types due to stack allocation and reduced garbage collection overhead.
Q: Can I use structs with Unity’s MonoBehaviour?
A: No, structs cannot inherit from MonoBehaviour. Use classes for Unity scripts that need to be attached to GameObjects.
In conclusion, understanding the differences between structs and classes is crucial for efficient game development in Unity. By choosing the right data structure for each situation, we can optimize our FPS game’s performance and maintain clean, organized code.