CSharp Foreach: Mastering Loops in Game Development
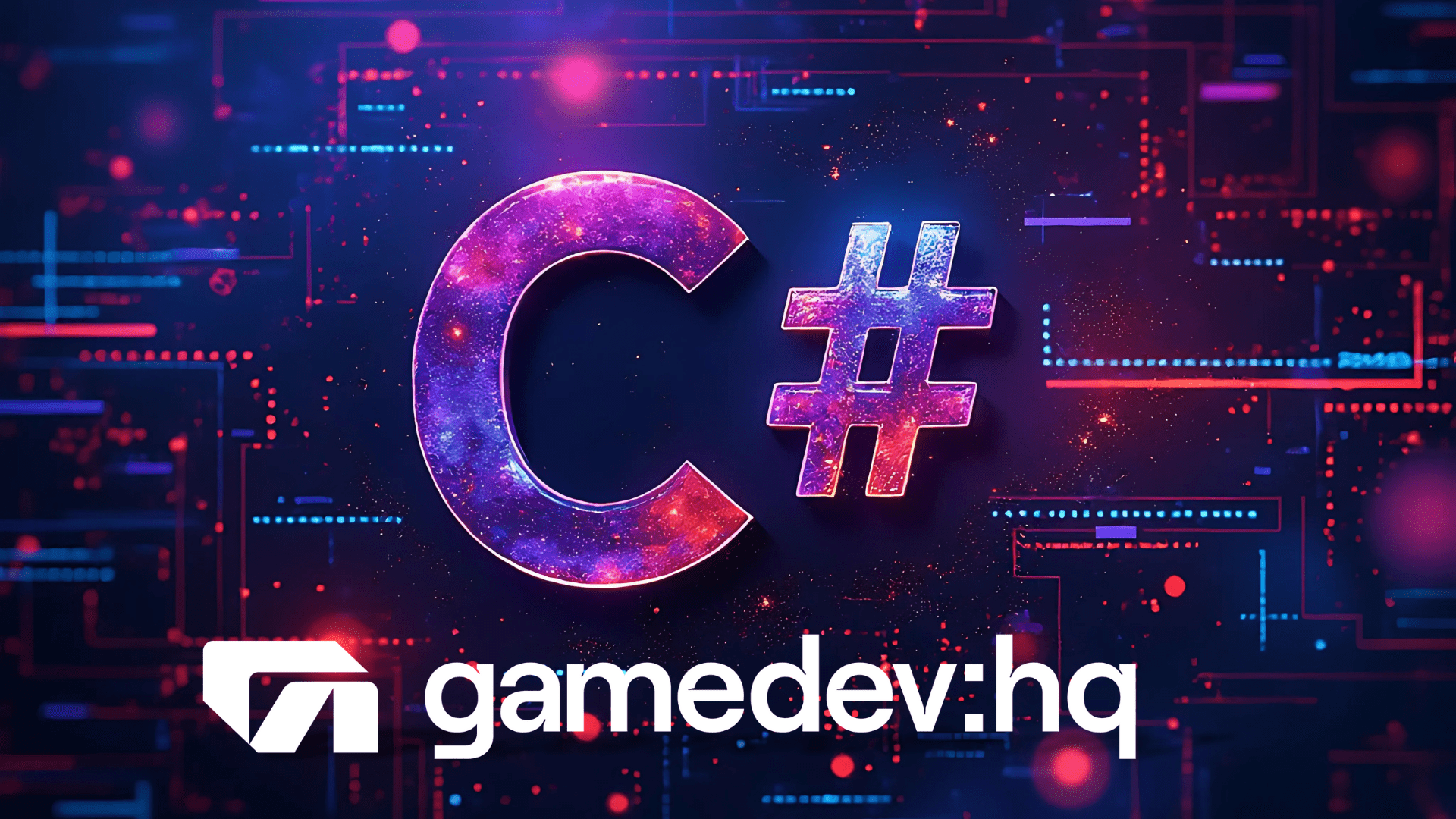
As a game developer, I’ve found that understanding loops is crucial for creating engaging gameplay mechanics. The foreach loop in C# is a powerful tool that I use frequently when working on various game projects. Let me walk you through how foreach works in C# and how it can enhance your game development process.
What is Foreach in C#?
Foreach is a loop structure in C# that allows you to iterate over elements in a collection without needing to manage an index or loop counter. It’s designed to make your code cleaner and less prone to errors when working with arrays, lists, and other enumerable objects.
Think of foreach like a conveyor belt in a platformer game. Each thing on the belt represents an item in your collection, and the loop processes each thing one by one as it moves along.
Basic Syntax of Foreach
Here’s the basic structure of a foreach loop in C#:
foreach (var item in collection)
{
// Code to execute for each item
}
In this syntax:
- ‘var’ is the type of the current item (C# can often infer this)
- ‘item’ is a variable that represents the current element in the iteration
- ‘collection’ is the array, list, or other enumerable object you’re looping through
Using Foreach with Arrays
Arrays are common in game development for storing things like inventory items, enemy types, or level layouts. Here’s how you might use foreach with an array:
string[] weapons = { "Sword", "Bow", "Axe", "Spear" };
foreach (string weapon in weapons)
{
Console.WriteLine($"Player equipped: {weapon}");
}
This code loops through each weapon in the array, printing a message for each one. It’s much simpler than using a traditional for loop, especially when you don’t need to know the index of each item.
Foreach with Lists
Lists are dynamic collections that can grow or shrink, making them useful for things like quest logs or active buffs on a player. Here’s an example using foreach with a List:
List<Enemy> enemies = GetEnemiesInRange();
foreach (Enemy enemy in enemies)
{
enemy.Attack(player);
}
This code iterates through all enemies in range and makes each one attack the player. The foreach loop makes this process straightforward and readable.
Nested Foreach Loops
Sometimes in game development, you need to work with 2D arrays or lists of lists. Nested foreach loops can handle these situations elegantly:
List<List<Tile>> levelMap = GenerateLevelMap();
foreach (List<Tile> row in levelMap)
{
foreach (Tile tile in row)
{
tile.Render();
}
}
This code might be used to render a 2D tile-based level in a game. The outer loop goes through each row, and the inner loop processes each tile in that row.
Foreach with LINQ
LINQ (Language Integrated Query) pairs beautifully with foreach loops. You can use LINQ to filter or transform your data before looping through it:
var highLevelEnemies = enemies.Where(e => e.Level > 10);
foreach (var enemy in highLevelEnemies)
{
enemy.DropRareItem();
}
This example filters for high-level enemies and then uses foreach to make each of them drop a rare item.
Performance Considerations
While foreach is convenient, it’s worth noting that in some cases, a traditional for loop might be slightly faster. However, the difference is often negligible, and the readability and safety of foreach usually outweigh tiny performance gains.
In game development, you might opt for a for loop when:
- You need the index of the current item
- You’re working with a fixed-size array and performance is critical
- You need to modify the collection while iterating (which foreach doesn’t allow)
Common Pitfalls to Avoid
- Modifying the Collection: You can’t add or remove items from the collection you’re iterating over with foreach. This can cause runtime errors.
- Performance with Value Types: When using foreach with value types (like structs), C# creates a copy of each item. This can be inefficient for large collections of complex structs.
- Null Collections: Always check if your collection is null before using foreach to avoid NullReferenceExceptions.
Foreach in Game Development Scenarios
Let’s look at some practical examples of how foreach can be used in game development:
- Updating Game Objects:
foreach (GameObject obj in activeObjects)
{
obj.Update(deltaTime);
}
- Collision Detection:
foreach (Collider collider in colliders)
{
if (player.Bounds.Intersects(collider.Bounds))
{
HandleCollision(player, collider);
}
}
- Applying Area of Effect (AoE) Damage:
foreach (Character character in charactersInRange)
{
character.TakeDamage(aoeDamage);
}
- Rendering UI Elements:
foreach (UIElement element in uiElements)
{
element.Draw(spriteBatch);
}
- Saving Game Progress:
foreach (InventoryItem item in player.Inventory)
{
SaveData.AddItem(item.ID, item.Quantity);
}
Advanced Foreach Techniques
- Using ‘var’ for Inference:
foreach (var enemy in enemies)
{
// C# infers the type of 'enemy'
}
- Deconstruction in Foreach:
foreach ((string name, int score) in leaderboard)
{
Console.WriteLine($"{name}: {score}");
}
- Using IEnumerable for Custom Collections:
public class EnemyWave : IEnumerable<Enemy>
{
private List<Enemy> enemies = new List<Enemy>();
public IEnumerator<Enemy> GetEnumerator()
{
return enemies.GetEnumerator();
}
IEnumerator IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
}
// Usage:
EnemyWave wave = new EnemyWave();
foreach (Enemy enemy in wave)
{
// Process each enemy
}
Foreach vs. For: When to Use Each
While foreach is often the go-to choice for its simplicity and safety, there are times when a traditional for loop might be more appropriate:
- When you need the index:
for (int i = 0; i < enemies.Count; i++)
{
Console.WriteLine($"Enemy {i + 1}: {enemies[i].Name}");
}
- When you need to iterate backwards:
for (int i = bullets.Count - 1; i >= 0; i--)
{
if (bullets[i].IsOffscreen)
{
bullets.RemoveAt(i);
}
}
- When you need to skip elements:
for (int i = 0; i < items.Count; i += 2)
{
// Process every other item
}
Optimizing Foreach Loops
- Avoid Unnecessary Allocations:
Instead of:
foreach (var item in myList.Where(x => x.IsActive))
Use:
foreach (var item in myList)
{
if (!item.IsActive) continue;
// Process active items
}
- Use ‘in’ keyword for readonly structs:
foreach (in Vector3 position in positions)
{
// Use position without copying
}
- Consider Parallel.ForEach for CPU-intensive operations:
Parallel.ForEach(heavyComputations, computation =>
{
computation.Process();
});
The foreach loop in C# is a versatile and powerful tool for game developers. Its simplicity makes it ideal for iterating over collections of game objects, inventory items, or any other enumerable data in your game. By mastering foreach, you can write cleaner, more maintainable code that’s less prone to errors.
Remember, like choosing the right weapon in an RPG, selecting the appropriate loop structure for each situation in your code can make a significant difference in your game’s performance and your productivity as a developer. Whether you’re managing a horde of enemies, updating UI elements, or processing game logic, foreach is often the right tool for the job.
As you continue to develop your C# skills, you’ll find that foreach becomes an indispensable part of your coding arsenal. Practice using it in various scenarios, and you’ll soon be wielding this powerful loop with the finesse of a skilled game programmer.