How to use Sin Cos in Game Dev – A Unity Math Tutorial
These mathematical tools are incredibly useful for creating smooth, cyclical movements in games. Let's explore how to harness their power to bring our game objects to life.
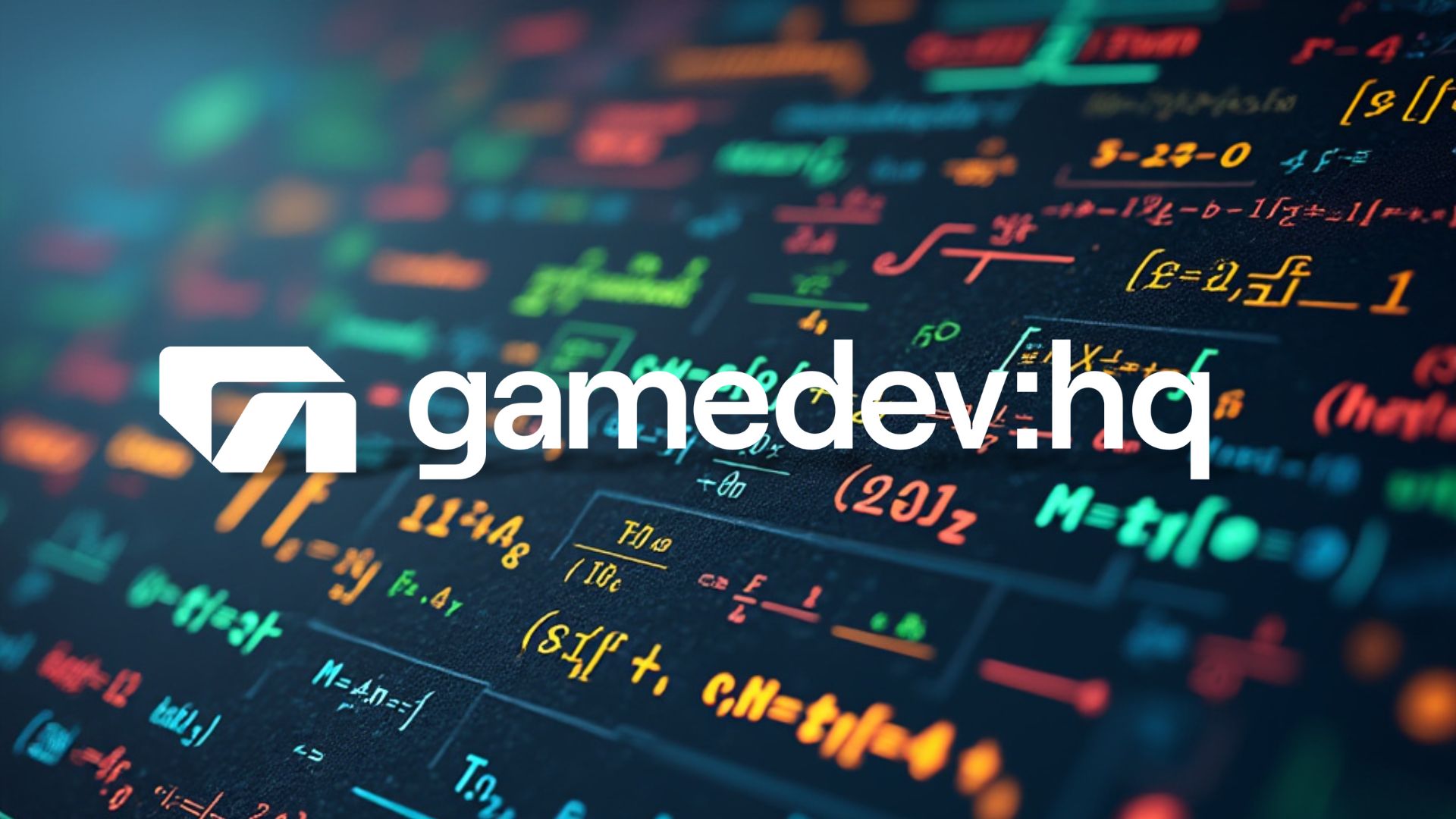
These mathematical tools are incredibly useful for creating smooth, cyclical movements in games. Let’s explore how to harness their power to bring our game objects to life.
If you prefer to watch, here’s my video on the topic:
Understanding Sine and Cosine
Sine and cosine are trigonometric functions that produce wave-like patterns. In Unity, we use them to create oscillating movements, which can be perfect for various game mechanics.
Sine Wave
A sine wave oscillates between -1 and 1 over time. It starts at 0, rises to 1, drops to -1, and then returns to 0. This pattern repeats indefinitely.
Cosine Wave
A cosine wave is similar to a sine wave but shifted 90 degrees ahead. It starts at 1, drops to -1, rises back to 1, and continues this cycle.
In game development, these waves can translate into smooth, repeating motions. Imagine a platformer where floating platforms move up and down – that’s a perfect use case for a sine wave!
Implementing Sine and Cosine in Unity
Let’s create a simple script to demonstrate how to use sine and cosine in Unity:
using UnityEngine;
public class SineCosineMovement : MonoBehaviour
{
[SerializeField] private float amplitude = 1f;
[SerializeField] private float frequency = 1f;
private void Update()
{
float x = transform.position.x;
float y = transform.position.y;
float z = transform.position.z;
// Sine wave for vertical movement
y = Mathf.Sin(Time.time * frequency) * amplitude;
// Cosine wave for horizontal movement
x = Mathf.Cos(Time.time * frequency) * amplitude;
transform.position = new Vector3(x, y, z);
}
}
In this script, we’re using Mathf.Sin()
and Mathf.Cos()
to create oscillating movements. The Time.time
parameter ensures our movement progresses with time.
Amplitude and Frequency
Two key concepts in working with sine and cosine are amplitude and frequency:
Amplitude
Amplitude determines the range of the oscillation. A higher amplitude means a wider range of movement.
Frequency
Frequency controls how quickly the oscillation occurs. A higher frequency results in faster movement.
By adjusting these values, we can create a variety of movement patterns. For instance, in a space shooter game, we could use this to create enemy ships that move in complex patterns, making them more challenging to hit.
Creating Circular Motion
One of the most interesting applications of sine and cosine is creating circular motion. When we use sine for vertical movement and cosine for horizontal movement simultaneously, we get a perfect circle.
void Update()
{
float x = Mathf.Cos(Time.time * frequency) * amplitude;
float y = Mathf.Sin(Time.time * frequency) * amplitude;
transform.position = new Vector3(x, y, transform.position.z);
}
This could be used in an RPG to create orbiting objects around the player, like a magical shield or floating crystals.
Practical Applications in Game Development
- Floating Objects: Use a sine wave to make objects hover or bob up and down.
- Swinging Obstacles: Apply sine or cosine to rotate objects, creating swinging pendulums or rotating platforms.
- Enemy Movement: Create enemies that move in wave-like patterns, making them more challenging to predict and defeat.
- Visual Effects: Use these functions to pulse the size or opacity of objects for attention-grabbing effects.
- Camera Shake: Implement a subtle camera shake effect by applying small sine wave movements to the camera position.
Advanced Techniques
As you become more comfortable with sine and cosine, you can explore more advanced techniques:
Combining Waves: Mix multiple sine or cosine waves with different frequencies and amplitudes to create complex motion patterns.
Phase Shifting: Introduce a phase shift to offset the starting point of your oscillation.
3D Movement: Extend the concept to three dimensions by applying sine and cosine to different axes.
By mastering these concepts, you’ll have powerful tools at your disposal for creating dynamic and engaging gameplay elements. Remember, the key to becoming proficient with these mathematical functions is experimentation. Don’t be afraid to play around with different values and combinations to see what interesting effects you can create.
In conclusion, sine and cosine functions are invaluable tools in a game developer’s arsenal. They allow us to create smooth, cyclical movements that can enhance gameplay, improve visual appeal, and add a layer of polish to our games. Whether you’re making a simple mobile game or a complex 3D world, understanding and applying these mathematical concepts will undoubtedly elevate your game development skills.