Learning Linq in C Sharp – an Introductory Guide
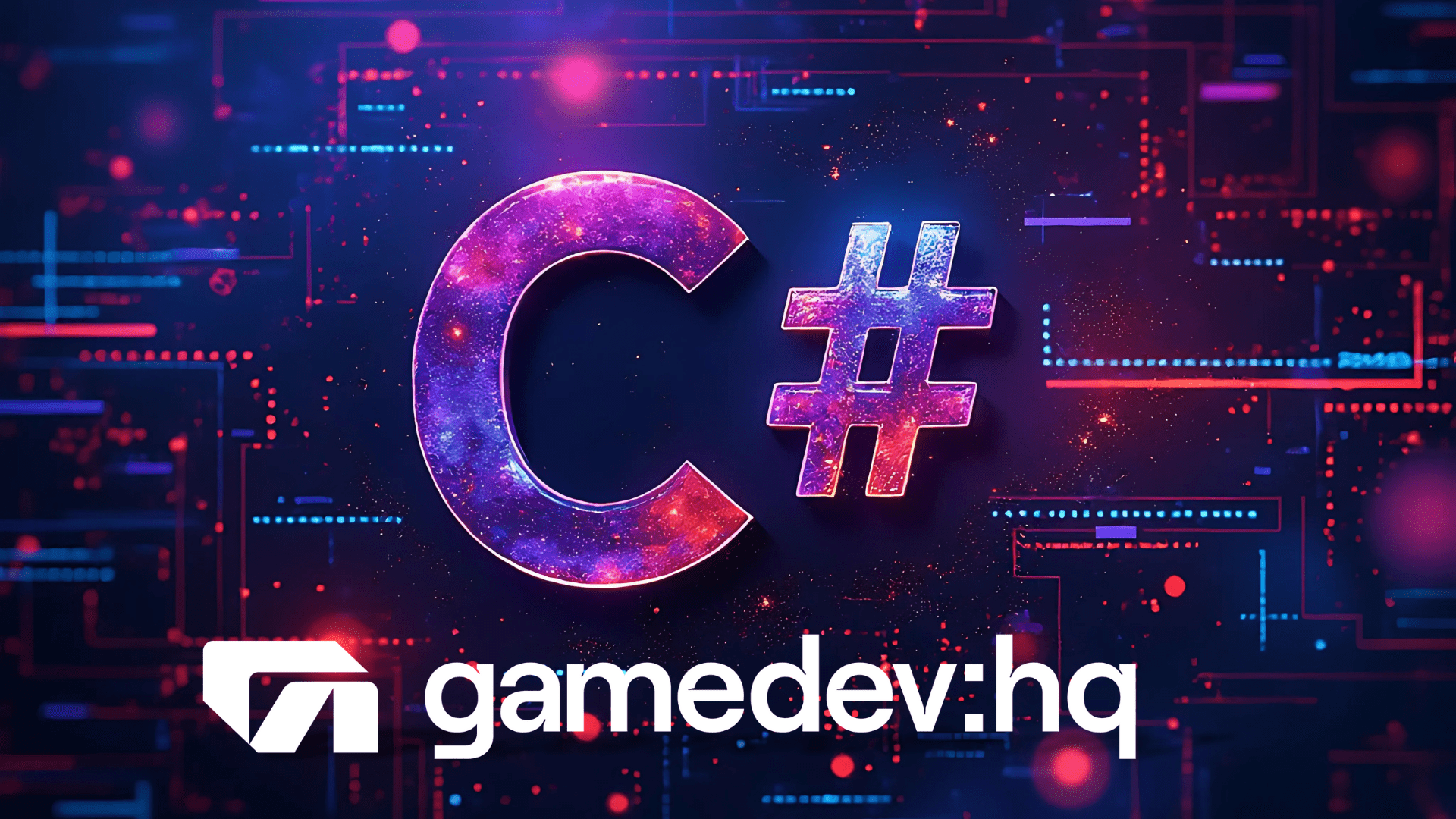
Let’s dive into LINQ in C#, a powerful feature that transforms how we handle data in our code. As a game developer, I’ve found LINQ invaluable for managing complex data structures, especially in RPGs where we often deal with large collections of items, characters, and quests.
What is LINQ?
LINQ, or Language Integrated Query, is a set of features in C# that extends its querying capabilities to various data sources. It provides a consistent way to work with data, whether it’s in-memory objects, databases, XML, or other formats.
Think of LINQ as a Swiss Army knife for data manipulation. In an RPG, you might use it to filter through your inventory, sort quest logs, or group enemies by type. It’s like having a magical spell that can sift through mountains of data in an instant.
Basic LINQ Syntax
LINQ queries often use a SQL-like syntax, making them intuitive for developers familiar with database queries. Here’s a simple example:
var highLevelCharacters = from character in partyMembers
where character.Level > 50
select character;
This query filters a collection of partyMembers
to find characters above level 50. It’s clean, readable, and powerful.
LINQ Method Syntax
While query syntax is expressive, method syntax offers more flexibility and is often preferred:
var highLevelCharacters = partyMembers.Where(c => c.Level > 50);
This achieves the same result as the previous example but uses lambda expressions, a cornerstone of LINQ’s power.
Common LINQ Operations
Filtering with Where
The Where
method lets you filter collections based on a condition:
var healers = characters.Where(c => c.Class == "Healer");
Ordering with OrderBy
OrderBy
sorts your data:
var sortedByLevel = characters.OrderBy(c => c.Level);
Selecting with Select
Select
transforms each element in a collection:
var characterNames = characters.Select(c => c.Name);
Grouping with GroupBy
GroupBy
organizes data into groups based on a key:
var charactersByClass = characters.GroupBy(c => c.Class);
LINQ and Game Development
In game development, LINQ shines in numerous scenarios. Let’s explore a few:
Inventory Management
Imagine an RPG inventory system. LINQ can help you quickly find items:
var healingPotions = inventory.Where(item => item.Type == ItemType.HealingPotion);
Or sort items by value:
var valuableLoot = inventory.OrderByDescending(item => item.Value).Take(5);
Quest System
LINQ can simplify quest management:
var availableQuests = allQuests.Where(q => q.Level <= player.Level && !q.IsCompleted);
Enemy AI
Use LINQ to find nearby enemies:
var nearbyEnemies = enemies.Where(e => Vector3.Distance(player.Position, e.Position) < 10f);
Performance Considerations
While LINQ is powerful, it’s important to use it judiciously, especially in performance-critical sections of your game. For large collections or frequently executed queries, traditional loops might be more efficient.
Deferred Execution
One of LINQ’s key features is deferred execution. When you write a LINQ query, it doesn’t execute immediately. Instead, it waits until you iterate over the results or call a method that forces execution (like ToList()
).
This can be both a blessing and a curse. It allows for efficient chaining of operations but can lead to unexpected behavior if not understood properly.
LINQ to SQL
LINQ isn’t limited to in-memory collections. LINQ to SQL allows you to query databases using the same syntax:
var highScores = from score in dbContext.Scores
where score.Value > 1000000
orderby score.Value descending
select score;
This query translates to SQL and executes on the database server, combining the power of LINQ with efficient database operations.
Advanced LINQ Techniques
Joining Data
LINQ provides powerful joining capabilities:
var playerItems = from player in players
join item in items on player.EquippedItemId equals item.Id
select new { PlayerName = player.Name, ItemName = item.Name };
This joins player data with their equipped items, creating a new anonymous type with the relevant information.
Aggregate Functions
LINQ includes several aggregate functions like Sum
, Average
, Min
, and Max
:
int totalExperience = characters.Sum(c => c.Experience);
double averageLevel = characters.Average(c => c.Level);
Custom Extensions
You can extend LINQ with your own methods:
public static class LinqExtensions
{
public static IEnumerable<T> WithinRange<T>(this IEnumerable<T> source,
Func<T, float> selector, Vector3 center, float range)
{
return source.Where(item => Vector3.Distance(center, selector(item)) <= range);
}
}
// Usage
var nearbyItems = items.WithinRange(i => i.Position, player.Position, 5f);
This custom extension method finds all items within a certain range of the player, demonstrating how LINQ can be tailored to specific game development needs.
Best Practices
- Use LINQ for readability when working with collections.
- Be aware of performance implications, especially with large datasets.
- Understand deferred execution and its implications.
- Combine LINQ with other C# features like async/await for efficient data processing.
- Use method syntax for complex queries and query syntax for simpler, more readable ones.
Conclusion
LINQ is a powerful tool in the C# developer’s arsenal, especially valuable in game development scenarios. It simplifies data manipulation tasks, making your code more readable and maintainable. Like a skilled mage in an RPG, mastering LINQ allows you to wield complex data spells with ease, transforming and filtering your game’s data effortlessly.
As you continue your journey in C# and game development, LINQ will prove to be an indispensable ally. Practice using it in various scenarios, from simple filtering to complex data transformations, and you’ll find your code becoming more expressive and powerful. Happy coding, and may your LINQ queries always return the data you seek!