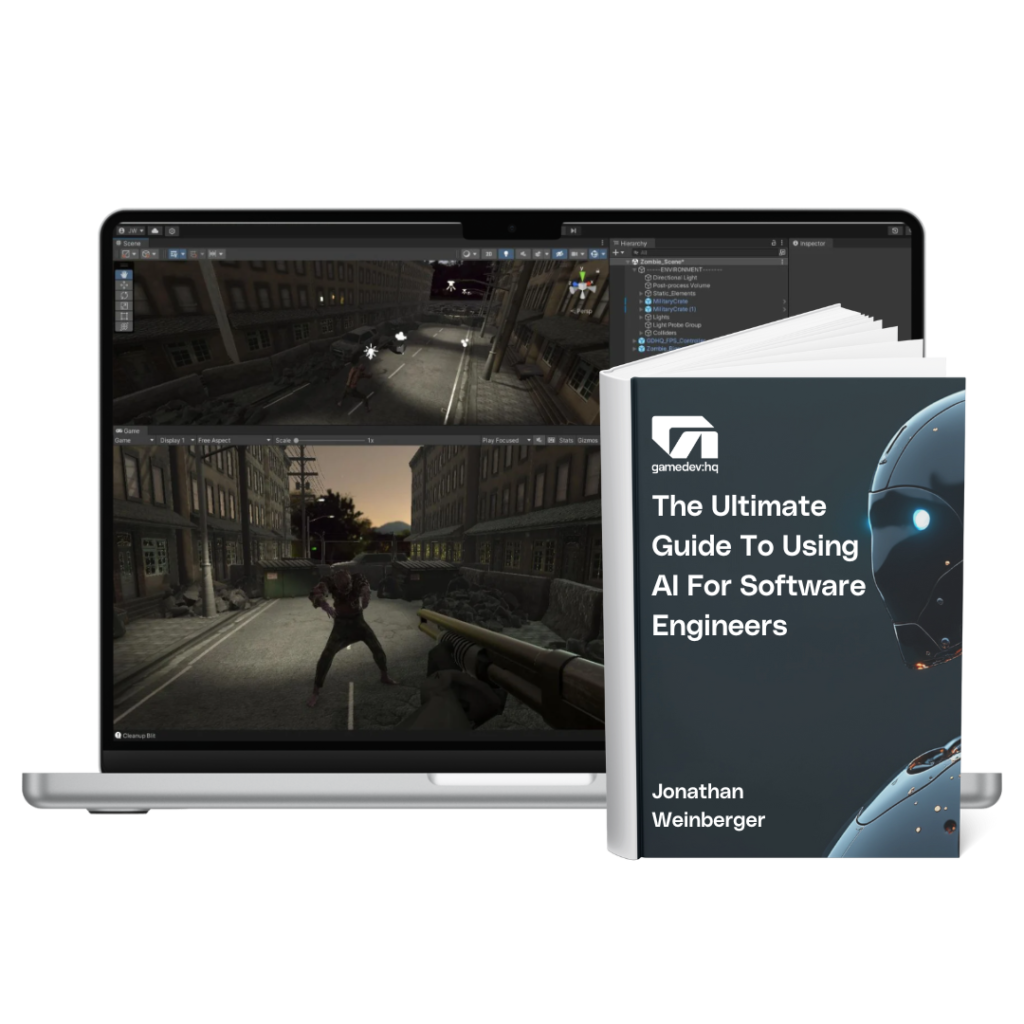
Mastering Logic Operators in C#: A Game Developer’s Guide
As a game developer, understanding logic operators is like learning the rules of engagement for your digital battlefield. These operators are the building blocks that allow your game to make decisions, compare values, and create intricate gameplay mechanics. Let’s dive into the world of logic operators in C# and see how they can level up your game development skills.
The Power of Comparison
Imagine you’re creating a first-person shooter (FPS) game. Your player’s health, ammo count, and score are all governed by logic operators. These silent sentinels constantly evaluate conditions, determining whether your character lives or dies, can fire a weapon, or has achieved a high score.
Let’s break down the most common logic operators and how they might apply in our FPS scenario:
Greater Than (>)
if (playerHealth > 0)
{
Debug.Log("Player is still alive!");
}
Less Than (<)
if (ammoCount < 10)
{
Debug.Log("Low ammo warning!");
}
Equal To (==)
if (enemiesDefeated == levelTarget)
{
Debug.Log("Level complete!");
}
Greater Than or Equal To (>=)
if (playerScore >= highScore)
{
Debug.Log("New high score!");
}
Less Than or Equal To (<=)
if (timeRemaining <= 0)
{
Debug.Log("Game over!");
}
Not Equal To (!=)
if (currentWeapon != "Sniper Rifle")
{
Debug.Log("Switch to sniper for this mission!");
}
Arithmetic in Action
Logic operators aren’t just for simple comparisons. They can involve arithmetic operations, allowing for more complex conditions:
if ((playerHealth + healthPack) > maxHealth)
{
playerHealth = maxHealth;
Debug.Log("Health maxed out!");
}
In this example, we’re checking if picking up a health pack would exceed the player’s maximum health, and if so, we cap it at the maximum.
The Order of Operations: PEMDAS
Remember PEMDAS (Parentheses, Exponents, Multiplication and Division, Addition and Subtraction) from math class? It’s crucial in programming too. Let’s calculate the average damage dealt in a round:
float averageDamage = (headshots + bodyshots + legshots) / 3f;
The parentheses ensure we sum up all the shots before dividing, giving us the correct average.
Logical AND (&&) and OR (||)
These operators allow us to combine conditions:
if (playerHealth > 0 && ammoCount > 0)
{
Debug.Log("Ready for combat!");
}
if (playerHealth <= 0 || timeRemaining <= 0)
{
Debug.Log("Game over!");
}
The AND operator (&&) requires both conditions to be true, while the OR operator (||) only needs one to be true.
The Power of NOT (!)
The NOT operator inverts a boolean condition:
bool isReloading = false;
if (!isReloading)
{
Debug.Log("Ready to fire!");
}
This is equivalent to if (isReloading == false)
.
Compound Assignment Operators
These operators combine arithmetic with assignment:
playerScore += killPoints; // Equivalent to: playerScore = playerScore + killPoints
ammoCount -= shotsFired; // Equivalent to: ammoCount = ammoCount - shotsFired
Ternary Operator
This compact operator can replace simple if-else statements:
string status = (playerHealth > 50) ? "Healthy" : "Wounded";
This assigns “Healthy” to status if playerHealth is greater than 50, and “Wounded” otherwise.
FAQs
Q: Why use ‘==’ for comparison instead of ‘=’?
A: The single ‘=’ is for assignment, while ‘==’ is for comparison. Using ‘=’ in an if statement would assign a value rather than compare it.
Q: Can I chain multiple conditions?
A: Yes, you can use && and || to chain as many conditions as needed. Just be mindful of readability.
Q: How do I check if a number is within a range?
A: You can use multiple conditions: if (value >= min && value <= max)
Q: What’s the difference between ‘&’ and ‘&&’?
A: ‘&&’ is a short-circuit operator. If the first condition is false, it doesn’t evaluate the second. ‘&’ always evaluates both sides.
Q: Can I use these operators with strings?
A: Some operators work with strings, like ‘==’ for equality and ‘+’ for concatenation. Others, like ‘<‘ and ‘>’, compare lexicographically.
Conclusion
Logic operators are the silent heroes of game development, working behind the scenes to create the dynamic, responsive experiences players love. Whether you’re checking if a player can level up, if an enemy is in range, or if it’s time to spawn a boss, these operators are your trusty tools.
As you continue your journey in game development, you’ll find yourself using these operators constantly. They’ll become second nature, allowing you to focus on the creative aspects of your game while these logical building blocks handle the nitty-gritty details.
Remember, practice makes perfect. Try implementing these operators in small projects or game mechanics. Before you know it, you’ll be crafting complex game logic with ease, bringing your virtual worlds to life one condition at a time.
Now, armed with the power of logic operators, go forth and create the next gaming masterpiece. The digital realm awaits your command!